c# .net3.5实现异步编程 实例
时间: 2023-07-27 17:19:14 浏览: 86
以下是一个使用.NET Framework 3.5实现异步编程的示例:
```csharp
using System;
using System.Threading;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Main thread started.");
// 使用委托和回调函数实现异步编程
Console.WriteLine("Using delegate and callback to implement asynchronous programming.");
MyDelegate myDelegate = new MyDelegate(DoSomething);
myDelegate.BeginInvoke(5000, new AsyncCallback(Callback), null);
// 使用线程池实现异步编程
Console.WriteLine("Using thread pool to implement asynchronous programming.");
ThreadPool.QueueUserWorkItem(new WaitCallback(DoSomethingThreadPool), 3000);
// 使用异步方法实现异步编程
Console.WriteLine("Using async method to implement asynchronous programming.");
DoSomethingAsync(2000);
Console.WriteLine("Main thread ended.");
Console.ReadLine();
}
// 定义委托和回调函数
public delegate void MyDelegate(int milliseconds);
public static void DoSomething(int milliseconds)
{
Console.WriteLine("DoSomething started.");
Thread.Sleep(milliseconds);
Console.WriteLine("DoSomething completed.");
}
public static void Callback(IAsyncResult ar)
{
Console.WriteLine("Callback started.");
MyDelegate myDelegate = (MyDelegate)ar.AsyncState;
myDelegate.EndInvoke(ar);
Console.WriteLine("Callback completed.");
}
// 使用线程池
public static void DoSomethingThreadPool(object state)
{
Console.WriteLine("DoSomethingThreadPool started.");
int milliseconds = (int)state;
Thread.Sleep(milliseconds);
Console.WriteLine("DoSomethingThreadPool completed.");
}
// 使用异步方法
public static async void DoSomethingAsync(int milliseconds)
{
Console.WriteLine("DoSomethingAsync started.");
await Task.Run(() => Thread.Sleep(milliseconds));
Console.WriteLine("DoSomethingAsync completed.");
}
}
```
该示例使用了三种不同的方法来实现异步编程:使用委托和回调函数、使用线程池、使用异步方法。在每种方法中,都使用Thread.Sleep方法模拟耗时操作。注意,在使用异步方法时,需要使用async和await关键字来标记异步方法和等待异步操作完成。
相关推荐
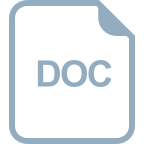
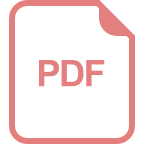
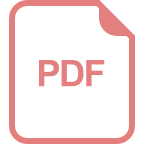














