输入一个标含标点符号的英文句子 统计输出句子中的单词个数 用Python打出来
时间: 2024-05-07 11:16:27 浏览: 11
Sure, I can help you with that. Here's the Python code to count the number of words in a sentence with punctuation:
```
sentence = input("Enter a sentence: ")
words = sentence.split()
print("Number of words in the sentence:", len(words))
```
You can copy and paste this code into your Python IDE or editor, and run it. Once you enter a sentence with punctuation, the code will split the sentence into words and count the number of words in the sentence.
相关问题
用Python实现输入一个包含标点符号的英文句子,统计输出句子中的单词个数
可以使用Python的split()函数将句子分割成单词列表,再使用len()函数计算列表的长度,即可得到句子中单词的个数。具体实现如下:
```python
sentence = input("请输入一个英文句子:")
words = sentence.split() # 使用split()函数将句子分割成单词列表
word_count = len(words) # 计算单词个数
print("句子中单词的个数为:", word_count)
```
需要注意的是,如果句子中有多余的空格或者标点符号,可能会导致单词列表中出现空字符串或者含有标点符号的字符串,需要在统计单词个数前对单词列表进行处理,去掉这些无用的字符串。可以使用正则表达式进行处理,具体实现如下:
```python
import re
sentence = input("请输入一个英文句子:")
words = re.findall(r'\b\w+\b', sentence) # 使用正则表达式匹配单词
word_count = len(words) # 计算单词个数
print("句子中单词的个数为:", word_count)
```
这里使用了正则表达式`\b\w+\b`来匹配单词,其中`\b`表示单词的边界,`\w+`表示匹配一个或多个字母数字字符。这样就可以去掉句子中的标点符号和空格,只统计单词的个数。
输入一个包含标点符号的英文句子,统计输出句子中的单词个数用Python怎么写
可以使用Python中的split()函数将句子按照空格分割成单词,并使用len()函数统计单词个数。示例代码如下:
```
sentence = input("请输入句子:")
words = sentence.split()
print("单词个数为:", len(words))
```
如果句子中包含其他标点符号,可以使用正则表达式或字符串处理函数去除标点符号。例如,可以使用re模块的sub()函数将句子中的标点符号替换为空格,然后再按照空格分割单词。示例代码如下:
```
import re
sentence = input("请输入句子:")
sentence = re.sub(r'[^\w\s]', ' ', sentence) # 将标点符号替换为空格
words = sentence.split()
print("单词个数为:", len(words))
```
相关推荐
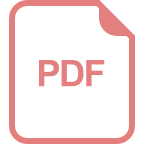
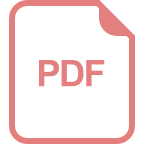
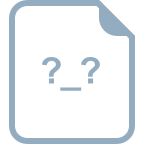












