ValueError: Image size of 630x193429 pixels is too large. It must be less than 2^16 in each direction. <Figure size 640x480 with 1 Axes>
时间: 2023-11-27 21:50:49 浏览: 130
这个错误的意思是你正在处理的图片大小超出了所允许的大小,必须小于2^16。你需要调整图片大小,使其小于这个限制。你可以使用Python的Pillow库来实现图片的缩放和调整大小。以下是一个示例代码,可以将图片大小调整为指定的大小:
```python
from PIL import Image
# 打开图片
img = Image.open("example.png")
# 调整大小
new_img = img.resize((800, 600))
# 保存图片
new_img.save("example_resized.png")
```
在上面的代码中,我们首先使用Pillow库打开一张图片,然后使用`resize()`方法将其大小调整为800x600,最后将新的图片保存到磁盘上。你可以根据需要将示例代码中的参数进行修改,以满足自己的需求。
相关问题
plt.text(-0.4, 500, 'frequency', horizontalalignment='center', size='x-small') 运行报错 ValueError: Image size of 630x193429 pixels is too large. It must be less than 2^16 in each direction. <Figure size 640x480 with 1 Axes>
这个错误是由于图片大小超过了限制。你可以尝试修改图片大小,或者调整文本的位置和大小,以适应图像大小限制。比如可以将文本位置向下移动一些,或者减小文本字体大小。代码示例:
```
plt.text(-0.4, 400, 'frequency', horizontalalignment='center', size='xx-small')
```
你可以根据具体情况适当调整文本位置和大小。如果还有问题,请告诉我具体的代码和数据,我可以帮你进一步排查。
ValueError: Image size of 1500x100000 pixels is too large. It must be less than 2^16 in each direction
这个错误提示说明你尝试显示一张尺寸非常大的图片,而 Python 中的 Pillow 库限制了图片的最大尺寸为 2^16(即 65536)像素。你需要对这张图片进行缩放,使其尺寸小于 65536 像素。你可以使用 Pillow 库或者其他图像处理库来缩放图片,比如:
```python
from PIL import Image
# 打开图片
img = Image.open('your_image.jpg')
# 获取原始大小
width, height = img.size
# 如果图片尺寸超出限制,进行缩放
if width > 65536 or height > 65536:
# 计算缩放比例
scale = min(65536/width, 65536/height)
# 缩放图片
img = img.resize((int(width*scale), int(height*scale)))
# 显示图片
img.show()
```
这样就可以避免这个错误了。
相关推荐
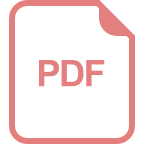
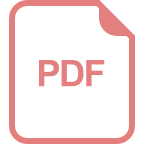
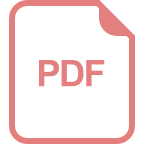












