写一段一维Capsnet网络代码keras
时间: 2023-12-27 09:02:47 浏览: 27
好的,以下是一段使用Keras实现的一维CapsNet网络代码:
```python
from keras import layers, models
class Capsule(layers.Layer):
def __init__(self, num_capsules, capsule_dim, routings=3, **kwargs):
super(Capsule, self).__init__(**kwargs)
self.num_capsules = num_capsules
self.capsule_dim = capsule_dim
self.routings = routings
def build(self, input_shape):
input_dim = input_shape[-1]
self.W = self.add_weight(shape=[input_dim, self.num_capsules * self.capsule_dim],
initializer='glorot_uniform',
name='W')
super(Capsule, self).build(input_shape)
def call(self, inputs):
inputs_expand = K.expand_dims(inputs, 2)
inputs_tiled = K.tile(inputs_expand, [1, 1, self.num_capsules, 1])
inputs_hat = K.map_fn(lambda x: K.batch_dot(x, self.W, [2, 1]), elems=inputs_tiled)
b = tf.zeros(shape=[K.shape(inputs_hat)[0], self.num_capsules, inputs.shape[1], 1])
for i in range(self.routings):
c = tf.nn.softmax(b, axis=1)
outputs = squash(K.batch_dot(c, inputs_hat, [2, 2]))
if i != self.routings - 1:
b += K.batch_dot(outputs, inputs_hat, [2, 3])
return K.reshape(outputs, [-1, self.num_capsules * self.capsule_dim])
def compute_output_shape(self, input_shape):
return tuple([None, self.num_capsules * self.capsule_dim])
def get_config(self):
config = {'num_capsules': self.num_capsules,
'capsule_dim': self.capsule_dim,
'routings': self.routings}
base_config = super(Capsule, self).get_config()
return dict(list(base_config.items()) + list(config.items()))
def squash(x, axis=-1):
s_squared_norm = K.sum(K.square(x), axis, keepdims=True)
scale = K.sqrt(s_squared_norm + K.epsilon())
return x / scale
def build_capsnet(input_shape, n_class, routings):
x = layers.Input(shape=input_shape)
conv1 = layers.Conv1D(filters=256, kernel_size=9, strides=1, padding='valid', activation='relu', name='conv1')(x)
primarycaps = layers.Conv1D(filters=256, kernel_size=9, strides=2, padding='valid', name='primarycaps')(conv1)
primarycaps = layers.BatchNormalization()(primarycaps)
primarycaps = layers.Activation('relu')(primarycaps)
primarycaps = layers.Reshape(target_shape=[-1, 8], name='primarycaps_reshape')(primarycaps)
digitcaps = Capsule(10, 16, routings=routings, name='digitcaps')(primarycaps)
out_caps = layers.Length(name='out_caps')(digitcaps)
y = layers.Input(shape=(n_class,))
masked_by_y = Mask()([digitcaps, y])
masked = Mask()(digitcaps)
x_recon = layers.Dense(512, activation='relu')(masked)
x_recon = layers.Dense(1024, activation='relu')(x_recon)
x_recon = layers.Dense(np.prod(input_shape), activation='sigmoid')(x_recon)
x_recon = layers.Reshape(target_shape=input_shape, name='out_recon')(x_recon)
return models.Model([x, y], [out_caps, x_recon])
```
这是一个使用Keras搭建的一维CapsNet网络,包含了Capsule和build_capsnet两个函数。Capsule函数是定义了一个Capsule层,build_capsnet函数则是搭建了一个完整的CapsNet网络。
相关推荐
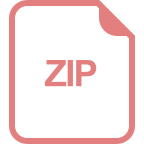
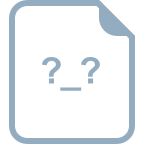
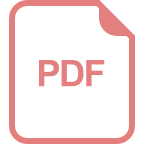










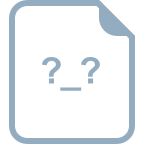