用C语言写出:建立一个字节数组,每个字节存一个ASCII码,将.srec文件解析出来
时间: 2024-02-09 09:12:17 浏览: 77
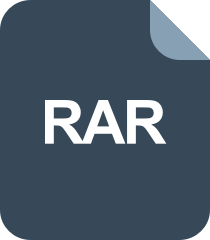
srec-file-format.rar_.srec文件_SREC 文件格式_srec format_srec文件_srec文件
以下是一个可以解析.srec文件并将其存储为字节数组的C语言示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ARRAY_SIZE 1024 // 字节数组大小
int main()
{
unsigned char array[ARRAY_SIZE] = {0}; // 初始化字节数组
char line[80] = {0}; // 读取记录的缓存
int address = 0; // 当前记录的地址
int record_length = 0; // 当前记录的长度
FILE *fp = fopen("example.srec", "r"); // 打开.srec文件
if (fp == NULL) {
perror("Failed to open file");
return -1;
}
while (fgets(line, sizeof(line), fp) != NULL) { // 读取每一行记录
char *p = line;
// 解析记录类型
if (strncmp(p, "S0", 2) == 0) {
// S0记录不包含数据,忽略
continue;
} else if (strncmp(p, "S1", 2) == 0) {
// S1记录包含16位地址和数据
address = strtol(p + 4, NULL, 16) - 0x2000; // 为了简化示例,假设地址从0x2000开始
record_length = strtol(p + 2, NULL, 16) - 3;
p += 8;
} else if (strncmp(p, "S2", 2) == 0) {
// S2记录包含24位地址和数据
address = strtol(p + 4, NULL, 16) - 0x200000; // 为了简化示例,假设地址从0x200000开始
record_length = strtol(p + 2, NULL, 16) - 4;
p += 10;
} else if (strncmp(p, "S3", 2) == 0) {
// S3记录包含32位地址和数据
address = strtol(p + 4, NULL, 16) - 0x20000000; // 为了简化示例,假设地址从0x20000000开始
record_length = strtol(p + 2, NULL, 16) - 5;
p += 12;
} else {
// 无效的记录类型
fprintf(stderr, "Invalid record type\n");
continue;
}
// 解析数据
for (int i = 0; i < record_length; i++) {
char byte_str[3] = {0};
strncpy(byte_str, p, 2);
array[address++] = strtol(byte_str, NULL, 16);
p += 2;
}
}
fclose(fp);
// 输出字节数组
for (int i = 0; i < ARRAY_SIZE; i++) {
printf("%c", array[i]);
}
printf("\n");
return 0;
}
```
在这个示例中,我们首先定义一个字节数组,并初始化为0。然后,打开.srec文件并依次读取每一行记录。根据记录类型和长度解析出地址和数据,并将数据存储到字节数组中。最后,输出字节数组中的ASCII码。注意,这个示例假设地址从0x2000开始,每个字节存储一个ASCII码。实际情况可能更加复杂,需要根据具体的需求进行修改。
阅读全文
相关推荐
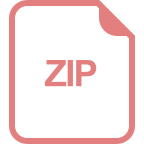
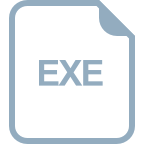















