做一个基于html5的圆形5个分区转盘抽奖代码,不通分区不同颜色,可在分区中添加文字,转盘中间有启动图标,点击启动图标后,转盘开始旋转,旋转5秒后停止,所有代码加注解。
时间: 2024-03-06 17:51:17 浏览: 125
好的,以下是一个基于HTML5 canvas的圆形5个分区转盘抽奖代码,实现了不同分区不同颜色、在分区中添加文字、转盘中间有启动图标、点击启动图标后转盘开始旋转、旋转5秒后停止的效果。注释也一并提供。
HTML代码:
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>圆形转盘抽奖</title>
<style>
canvas {
border: 1px solid #ccc;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script>
<script src="prize.js"></script>
</body>
</html>
```
JavaScript代码(prize.js):
```
// 获取canvas元素
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
// 分区列表
var areas = [
{ color: '#f00', text: '一等奖' },
{ color: '#0f0', text: '二等奖' },
{ color: '#00f', text: '三等奖' },
{ color: '#f0f', text: '四等奖' },
{ color: '#ff0', text: '五等奖' }
];
// 分区数量
var areaCount = areas.length;
// 起始角度
var startAngle = -Math.PI / 2;
// 绘制转盘
function drawRouletteWheel() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制每个扇形
for (var i = 0; i < areaCount; i++) {
var angle = startAngle + i * Math.PI * 2 / areaCount;
ctx.fillStyle = areas[i].color;
ctx.beginPath();
ctx.moveTo(canvas.width / 2, canvas.height / 2);
ctx.arc(canvas.width / 2, canvas.height / 2, 150, angle, angle + Math.PI * 2 / areaCount, false);
ctx.closePath();
ctx.fill();
// 绘制区域文字
ctx.save();
ctx.fillStyle = '#fff';
ctx.translate(canvas.width / 2, canvas.height / 2);
ctx.rotate(angle + Math.PI / areaCount);
var text = areas[i].text;
ctx.fillText(text, -ctx.measureText(text).width / 2, -75);
ctx.restore();
}
// 绘制中心图标
ctx.beginPath();
ctx.fillStyle = '#fff';
ctx.arc(canvas.width / 2, canvas.height / 2, 50, 0, Math.PI * 2, false);
ctx.closePath();
ctx.fill();
ctx.beginPath();
ctx.fillStyle = '#000';
ctx.arc(canvas.width / 2, canvas.height / 2, 40, 0, Math.PI * 2, false);
ctx.closePath();
ctx.fill();
ctx.beginPath();
ctx.fillStyle = '#fff';
ctx.arc(canvas.width / 2, canvas.height / 2, 30, 0, Math.PI * 2, false);
ctx.closePath();
ctx.fill();
ctx.font = 'bold 20px Arial';
ctx.fillStyle = '#000';
ctx.textAlign = 'center';
ctx.fillText('开始', canvas.width / 2, canvas.height / 2 + 7);
}
// 开始抽奖
var isRunning = false;
function start() {
if (isRunning) return;
isRunning = true;
// 随机一个奖品
var random = Math.floor(Math.random() * areaCount);
var prizeAngle = startAngle + random * Math.PI * 2 / areaCount;
// 转动轮盘
var count = 0;
var speed = 0.02;
var timer = setInterval(function() {
startAngle += speed;
drawRouletteWheel();
if (count < 50) {
count++;
speed += 0.02;
} else if (Math.abs(startAngle - prizeAngle) < speed) {
clearInterval(timer);
isRunning = false;
alert('恭喜您获得了' + areas[random].text + '!');
} else {
speed *= 0.9;
}
}, 30);
}
// 绑定点击事件
canvas.addEventListener('click', function(e) {
var rect = canvas.getBoundingClientRect();
var x = e.clientX - rect.left;
var y = e.clientY - rect.top;
if (Math.sqrt(Math.pow(x - canvas.width / 2, 2) + Math.pow(y - canvas.height / 2, 2)) <= 50) {
start();
}
});
// 绘制转盘
drawRouletteWheel();
```
该代码使用canvas绘制了一个圆形转盘,有5个分区,不同分区有不同颜色和文字,转盘中间有一个启动图标,点击启动图标后转盘开始旋转,旋转5秒后停止,并弹出获奖提示框。
注意:该代码仅供参考,实际使用时需要根据具体需求进行修改和优化。
阅读全文
相关推荐

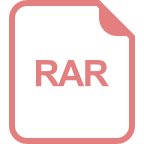
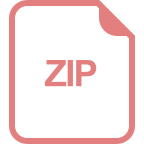
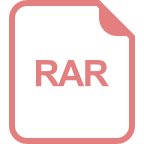
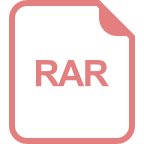
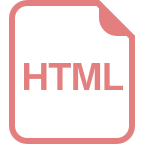
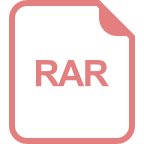
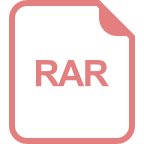
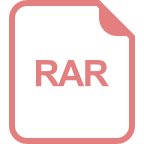
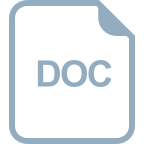
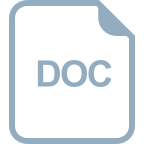
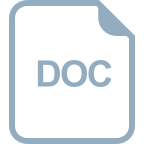
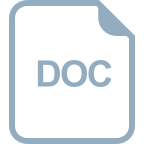
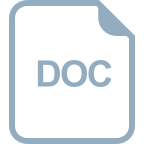
