设置一个日期类,用重载运算符比较日期大小
时间: 2024-02-24 11:57:07 浏览: 69
好的,下面是一个简单的日期类的实现,包括比较运算符的重载:
```cpp
#include <iostream>
class Date {
public:
Date(int year, int month, int day)
: year_(year), month_(month), day_(day) {}
bool operator <(const Date& other) const {
if (year_ < other.year_) {
return true;
} else if (year_ == other.year_) {
if (month_ < other.month_) {
return true;
} else if (month_ == other.month_) {
return day_ < other.day_;
}
}
return false;
}
bool operator >(const Date& other) const {
return other < *this;
}
private:
int year_;
int month_;
int day_;
};
int main() {
Date d1(2021, 7, 1);
Date d2(2022, 1, 1);
if (d1 < d2) {
std::cout << "d1 is earlier than d2" << std::endl;
} else if (d1 > d2) {
std::cout << "d1 is later than d2" << std::endl;
} else {
std::cout << "d1 is the same as d2" << std::endl;
}
return 0;
}
```
在上面的代码中,我们重载了 `<` 和 `>` 运算符,用来比较两个日期的大小。比较的顺序是:先比较年份,如果年份相同再比较月份,如果月份也相同再比较日期。这样我们就可以直接使用 `<` 和 `>` 运算符来比较两个日期的大小了。
阅读全文
相关推荐
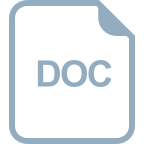
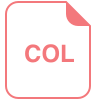
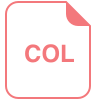
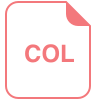
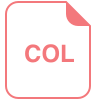
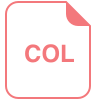
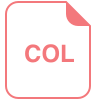
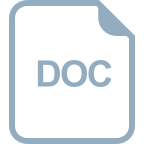
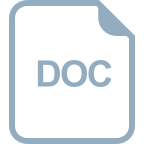
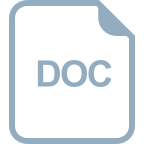
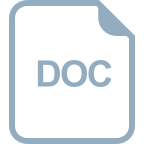

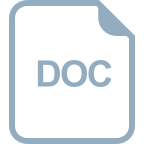
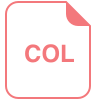
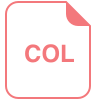
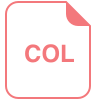

