声明一个People(人员)类,具有属性如下:number(编号)、name(姓名)、sex(性别)、birthday(出生日期)、id(身份证号)。其中“出生日期”是一个子对象(请自行设计日期类)。用成员函数实现对人员信息的录入和显示。在主函数中定义People类的对象数组,录入数据并显示。同时为People重载运算符“==”,运算符“==”为判断两个People对应的id属性是否相同
时间: 2024-01-15 20:02:45 浏览: 248
好的,我了解了您的要求。下面是所需的代码:
```
#include <iostream>
#include <string>
using namespace std;
class Date {
private:
int year, month, day;
public:
Date(int y = 0, int m = 0, int d = 0) {
year = y;
month = m;
day = d;
}
void setDate(int y, int m, int d) {
year = y;
month = m;
day = d;
}
void showDate() {
cout << year << "/" << month << "/" << day;
}
};
class People {
private:
int number;
string name;
string sex;
Date birthday;
string id;
public:
People() {}
People(int n, string na, string s, Date b, string i) {
number = n;
name = na;
sex = s;
birthday = b;
id = i;
}
void setInfo(int n, string na, string s, Date b, string i) {
number = n;
name = na;
sex = s;
birthday = b;
id = i;
}
void showInfo() {
cout << "编号:" << number << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:";
birthday.showDate();
cout << endl;
cout << "身份证号:" << id << endl;
}
bool operator == (const People& p) const {
return id == p.id;
}
};
int main() {
const int n = 3; // 定义对象数组大小
People people[n]; // 定义对象数组
int num;
string name, sex, id;
int year, month, day;
for (int i = 0; i < n; i++) {
cout << "请输入第" << i+1 << "个人的信息:" << endl;
cout << "编号:";
cin >> num;
cout << "姓名:";
cin >> name;
cout << "性别:";
cin >> sex;
cout << "出生日期(年/月/日):";
cin >> year >> month >> day;
Date date(year, month, day);
cout << "身份证号:";
cin >> id;
people[i].setInfo(num, name, sex, date, id);
}
cout << "录入的人员信息为:" << endl;
for (int i = 0; i < n; i++) {
people[i].showInfo();
}
// 判断两个People对象的id属性是否相同
if (people[0] == people[1]) {
cout << "第1个人和第2个人的身份证号相同" << endl;
}
else {
cout << "第1个人和第2个人的身份证号不同" << endl;
}
return 0;
}
```
您可以在本地编译运行此代码,以查看结果。
阅读全文
相关推荐
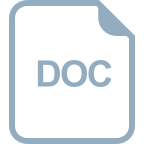
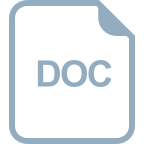
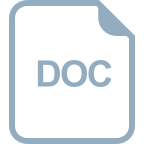
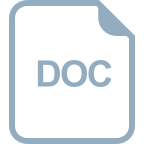
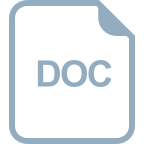


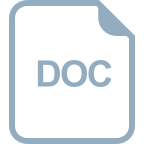
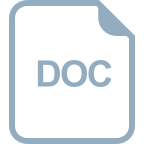
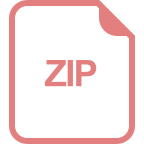