用python实现如下内容:将DDPG的训练过程改为多进程训练
时间: 2023-09-10 17:05:15 浏览: 63
下面是一个简单的使用Python实现DDPG算法的多进程训练的示例代码:
```python
import multiprocessing as mp
import numpy as np
import tensorflow as tf
from ddpg import DDPG
class DDPGWorker(mp.Process):
def __init__(self, worker_id, env, state_dim, action_dim, action_bound, buffer_size, batch_size, actor_lr, critic_lr):
super(DDPGWorker, self).__init__()
self.worker_id = worker_id
self.env = env
self.state_dim = state_dim
self.action_dim = action_dim
self.action_bound = action_bound
self.buffer_size = buffer_size
self.batch_size = batch_size
self.actor_lr = actor_lr
self.critic_lr = critic_lr
def run(self):
tf.compat.v1.disable_eager_execution()
with tf.compat.v1.Session() as sess:
ddpg = DDPG(self.state_dim, self.action_dim, self.action_bound, self.buffer_size, self.batch_size, self.actor_lr, self.critic_lr)
state = self.env.reset()
while True:
action = ddpg.choose_action(state)
next_state, reward, done, _ = self.env.step(action)
ddpg.store_transition(state, action, reward, next_state, done)
if ddpg.buffer_size >= self.batch_size:
ddpg.learn()
if done:
state = self.env.reset()
else:
state = next_state
class DDPGMultiWorker:
def __init__(self, num_workers, env, state_dim, action_dim, action_bound, buffer_size, batch_size, actor_lr, critic_lr):
self.num_workers = num_workers
self.env = env
self.state_dim = state_dim
self.action_dim = action_dim
self.action_bound = action_bound
self.buffer_size = buffer_size
self.batch_size = batch_size
self.actor_lr = actor_lr
self.critic_lr = critic_lr
def run(self):
workers = []
for i in range(self.num_workers):
worker = DDPGWorker(i, self.env, self.state_dim, self.action_dim, self.action_bound, self.buffer_size, self.batch_size, self.actor_lr, self.critic_lr)
workers.append(worker)
for worker in workers:
worker.start()
for worker in workers:
worker.join()
if __name__ == '__main__':
env = gym.make('Pendulum-v0')
state_dim = env.observation_space.shape[0]
action_dim = env.action_space.shape[0]
action_bound = env.action_space.high
buffer_size = 100000
batch_size = 64
actor_lr = 0.001
critic_lr = 0.002
num_workers = 4
ddpg_multi_worker = DDPGMultiWorker(num_workers, env, state_dim, action_dim, action_bound, buffer_size, batch_size, actor_lr, critic_lr)
ddpg_multi_worker.run()
```
在这个示例中,我们使用了Python的multiprocessing库来实现DDPG算法的多进程训练。首先,我们定义了一个DDPGWorker类,它是一个进程,负责收集经验数据和更新策略。然后,我们定义了一个DDPGMultiWorker类,它是一个主进程,负责协调不同的DDPGWorker进程。最后,我们创建了num_workers个DDPGWorker进程,并让它们运行DDPG算法的训练过程。注意,在DDPGWorker进程中,我们使用tensorflow.compat.v1.Session()而不是普通的tensorflow.Session(),以支持多进程训练。
总的来说,多进程训练是一种有效的方式来加速DDPG算法的训练过程,可以充分利用现代计算机的多核处理能力,提高训练效率和性能。
相关推荐
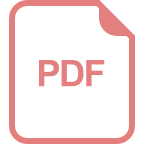
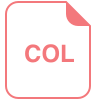
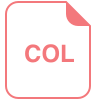
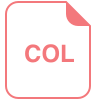
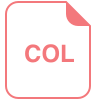
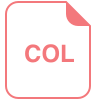









