arduino怎么将串口数据传到mysql
时间: 2024-05-04 10:20:23 浏览: 16
要将Arduino串口数据传输到MySQL,需要以下步骤:
1. 在计算机上安装MySQL数据库并启动MySQL服务。
2. 在MySQL数据库中创建一个数据库和一个表来存储串口数据。
3. 在Arduino IDE中编写一个程序,该程序将从串口读取数据并将其发送到计算机上的MySQL数据库。
4. 在计算机上编写一个程序来接收来自Arduino的串口数据并将其插入到MySQL表中。
以下是一个简单的示例程序,它从Arduino串口读取数据并将其发送到计算机上的MySQL数据库:
```c++
#include <SoftwareSerial.h>
#include <MySQL_Connection.h>
#include <MySQL_Cursor.h>
SoftwareSerial mySerial(10, 11); // RX, TX
char buffer[100];
int index = 0;
IPAddress server_addr(192,168,1,100); // IP address of the MySQL server
char user[] = "root"; // MySQL username
char password[] = "mypassword"; // MySQL password
MySQL_Connection conn((Client *)&mySerial);
void setup() {
Serial.begin(9600);
mySerial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
if (conn.connect(server_addr, 3306, user, password)) {
Serial.println("Connected to MySQL server");
} else {
Serial.println("Connection failed");
}
delay(1000);
}
void loop() {
if (mySerial.available()) {
char c = mySerial.read();
buffer[index++] = c;
if (c == '\n') {
buffer[index] = '\0';
index = 0;
Serial.print("Received data: ");
Serial.println(buffer);
insertData(buffer);
}
}
}
void insertData(char* data) {
MySQL_Cursor *cur_mem = new MySQL_Cursor(&conn);
char sql_query[255];
sprintf(sql_query, "INSERT INTO mytable (data) VALUES ('%s')", data);
cur_mem->execute(sql_query);
delete cur_mem;
}
```
请注意,此示例程序假定您已经在MySQL服务器上创建了一个名为“mytable”的表,并且该表包含一个名为“data”的列,以存储串口数据。
此外,您还需要在计算机上编写一个程序来接收来自Arduino的串口数据并将其插入到MySQL表中。这可以使用您选择的编程语言和MySQL客户端库完成。例如,您可以使用Python和PyMySQL库编写一个简单的程序来完成此操作。以下是一个示例程序:
```python
import serial
import pymysql
ser = serial.Serial('/dev/ttyACM0', 9600) # replace with your serial port
conn = pymysql.connect(host='localhost', port=3306, user='root', password='mypassword', database='mydatabase')
cur = conn.cursor()
while True:
data = ser.readline().strip()
print("Received data: ", data)
cur.execute("INSERT INTO mytable (data) VALUES (%s)", (data.decode('utf-8')))
conn.commit()
```
请注意,此示例程序假定您已经在MySQL服务器上创建了一个名为“mytable”的表,并且该表包含一个名为“data”的列,以存储串口数据。此外,您还需要根据您的实际设置更改程序中的串口和MySQL连接参数。
相关推荐
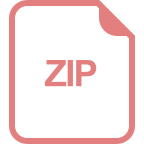
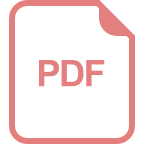
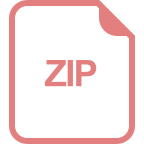














