举三个c语言代码帮忙理解指针
时间: 2023-07-24 13:44:14 浏览: 53
好的,以下是三个C语言代码来帮助理解指针:
1. 使用指针交换两个变量的值
```c
#include <stdio.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 10, y = 20;
printf("Before swap: x = %d, y = %d\n", x, y);
swap(&x, &y);
printf("After swap: x = %d, y = %d\n", x, y);
return 0;
}
```
这个代码演示了如何使用指针交换两个变量的值。`swap`函数接收两个指针作为参数,然后通过指针来访问变量并进行交换。
2. 使用指针遍历数组
```c
#include <stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
int *p = arr;
for (int i = 0; i < 5; i++) {
printf("%d ", *p);
p++;
}
return 0;
}
```
这个代码演示了如何使用指针遍历数组。`p`指针指向数组的第一个元素,然后使用循环依次访问每个元素并打印出来。
3. 使用指针作为函数参数
```c
#include <stdio.h>
void changeValue(int *p) {
*p = 20;
}
int main() {
int x = 10;
printf("Before change: x = %d\n", x);
changeValue(&x);
printf("After change: x = %d\n", x);
return 0;
}
```
这个代码演示了如何使用指针作为函数参数。`changeValue`函数接收一个指针作为参数,然后通过指针来访问变量并修改其值。在`main`函数中,我们将`x`的地址传递给函数,并在函数中修改`x`的值。最后,我们打印`x`的值来确认它是否已经被修改。
相关推荐
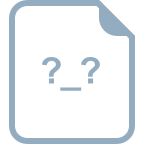
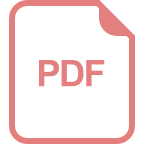
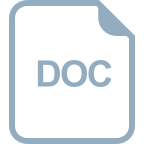














