用c++写学生选课系统
时间: 2023-08-04 16:26:46 浏览: 111
好的,下面是一个简单的 C++ 学生选课系统的实现:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Course {
private:
string name;
int id;
int capacity;
int enrolled;
public:
Course(const string& n, int i, int c) : name(n), id(i), capacity(c), enrolled(0) {}
int getId() const { return id; }
string getName() const { return name; }
int getCapacity() const { return capacity; }
int getEnrolled() const { return enrolled; }
bool isFull() const { return enrolled >= capacity; }
void enroll() { enrolled++; }
void drop() { enrolled--; }
};
class Student {
private:
string name;
int id;
vector<Course*> courses;
public:
Student(const string& n, int i) : name(n), id(i) {}
int getId() const { return id; }
string getName() const { return name; }
vector<Course*> getCourses() const { return courses; }
void enroll(Course* c) { courses.push_back(c); c->enroll(); }
void drop(Course* c) { courses.erase(find(courses.begin(), courses.end(), c)); c->drop(); }
};
class CourseManager {
private:
vector<Course> courses;
public:
void addCourse(const string& n, int i, int c) { courses.emplace_back(n, i, c); }
void removeCourse(int id) { courses.erase(find_if(courses.begin(), courses.end(), [id](const Course& c) { return c.getId() == id; })); }
vector<Course> getCourses() const { return courses; }
Course* findCourse(int id) {
for (auto& c : courses) {
if (c.getId() == id) {
return &c;
}
}
return nullptr;
}
};
class StudentManager {
private:
vector<Student> students;
public:
void addStudent(const string& n, int i) { students.emplace_back(n, i); }
void removeStudent(int id) { students.erase(find_if(students.begin(), students.end(), [id](const Student& s) { return s.getId() == id; })); }
vector<Student> getStudents() const { return students; }
Student* findStudent(int id) {
for (auto& s : students) {
if (s.getId() == id) {
return &s;
}
}
return nullptr;
}
};
void printCourses(const CourseManager& cm) {
cout << "Available courses:" << endl;
for (auto& c : cm.getCourses()) {
cout << c.getName() << " (ID: " << c.getId() << ", Capacity: " << c.getCapacity() << ", Enrolled: " << c.getEnrolled() << ")" << endl;
}
cout << endl;
}
void printStudents(const StudentManager& sm) {
cout << "Enrolled students:" << endl;
for (auto& s : sm.getStudents()) {
cout << s.getName() << " (ID: " << s.getId() << ", Courses: ";
auto courses = s.getCourses();
if (courses.empty()) {
cout << "none";
} else {
for (auto& c : courses) {
cout << c->getName() << " (ID: " << c->getId() << "), ";
}
cout << "\b\b"; // 删除最后的逗号和空格
}
cout << ")" << endl;
}
cout << endl;
}
int main() {
CourseManager cm;
StudentManager sm;
cm.addCourse("Math", 1, 30);
cm.addCourse("English", 2, 25);
cm.addCourse("Computer Science", 3, 20);
sm.addStudent("Alice", 1001);
sm.addStudent("Bob", 1002);
printCourses(cm);
printStudents(sm);
// Alice 选 Math 和 English,Bob 选 Math 和 Computer Science
auto math = cm.findCourse(1);
auto english = cm.findCourse(2);
auto cs = cm.findCourse(3);
auto alice = sm.findStudent(1001);
auto bob = sm.findStudent(1002);
alice->enroll(math);
alice->enroll(english);
bob->enroll(math);
bob->enroll(cs);
printCourses(cm);
printStudents(sm);
// Bob 退选 Math
bob->drop(math);
printCourses(cm);
printStudents(sm);
return 0;
}
```
在上面的代码中,我们定义了 `Course`、`Student`、`CourseManager` 和 `StudentManager` 四个类,分别表示课程、学生、课程管理器和学生管理器。课程类中包含了课程名称、课程 ID、课程容量和已选人数等私有成员变量,以及选课和退课方法等公有方法。学生类中包含了学生姓名、学生 ID 和已选课程等私有成员变量,以及选课和退课方法等公有方法。课程管理器中包含了课程列表等私有成员变量,以及添加课程、删除课程、获取课程列表和查找课程等公有方法。学生管理器中包含了学生列表等私有成员变量,以及添加学生、删除学生、获取学生列表和查找学生等公有方法。
在 `main()` 函数中,我们首先创建了一个课程管理器和一个学生管理器,并添加了一些课程和学生。然后,我们调用了 `printCourses()` 和 `printStudents()` 函数,分别输出了可选课程和已选课程的信息。接着,我们让 Alice 选了 Math 和 English 两门课,Bob 选了 Math 和 Computer Science 两门课,并再次调用了 `printCourses()` 和 `printStudents()` 函数,输出了更新后的课程和学生信息。最后,我们让 Bob 退选了 Math 课,并再次调用了 `printCourses()` 和 `printStudents()` 函数,输出了最终的课程和学生信息。
阅读全文
相关推荐






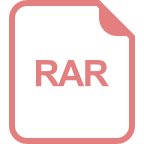




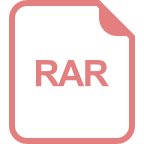



