用c++类编写学生选课系统程序
时间: 2023-08-28 21:06:48 浏览: 39
好的,我可以帮你解答这个问题。以下是一个简单的学生选课系统的C++类程序示例:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Course {
private:
string name;
int id;
int capacity;
int enrolled;
public:
Course(string name, int id, int capacity) {
this->name = name;
this->id = id;
this->capacity = capacity;
this->enrolled = 0;
}
string get_name() {
return this->name;
}
int get_id() {
return this->id;
}
int get_capacity() {
return this->capacity;
}
int get_enrolled() {
return this->enrolled;
}
bool add_student() {
if (this->enrolled < this->capacity) {
this->enrolled++;
return true;
} else {
return false;
}
}
};
class Student {
private:
string name;
int id;
vector<Course*> courses;
public:
Student(string name, int id) {
this->name = name;
this->id = id;
}
string get_name() {
return this->name;
}
int get_id() {
return this->id;
}
void enroll(Course* course) {
if (course->add_student()) {
this->courses.push_back(course);
cout << "Enrolled " << course->get_name() << endl;
} else {
cout << "Course is full: " << course->get_name() << endl;
}
}
void list_courses() {
cout << "Courses for " << this->name << ":" << endl;
for (int i = 0; i < this->courses.size(); i++) {
cout << "- " << this->courses[i]->get_name() << endl;
}
}
};
int main() {
Course c1("Math", 1, 30);
Course c2("Science", 2, 20);
Student s1("Alice", 1);
Student s2("Bob", 2);
s1.enroll(&c1);
s1.enroll(&c2);
s2.enroll(&c1);
s1.list_courses();
s2.list_courses();
return 0;
}
```
该程序包含两个类:`Course`和`Student`。`Course`类表示一个课程,包含名称、ID、容量和已选学生数。`Student`类表示一个学生,包含姓名、ID和所选的课程列表。
在主函数中,我们创建两个课程和两个学生,并让学生选择课程。学生选择课程时,我们将其添加到课程的选课列表中,并打印出成功或失败的消息。在最后,我们列出了每个学生所选的课程列表。
这只是一个简单的示例,实际的学生选课系统需要更多的功能和错误处理。但是,这应该可以帮助你开始编写自己的程序。
相关推荐
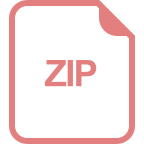
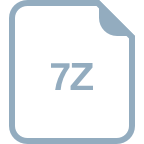
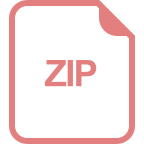















