用c++写一个桶排序
时间: 2024-02-18 18:55:58 浏览: 82
桶排序是一种基于非比较的排序方式,时间复杂度O(n),因此它是是属于一种“线性排序”。下面是用C++实现桶排序的代码:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void bucketSort(vector<int>& nums, int maxVal) {
vector<int> bucket(maxVal + 1, 0);
for (int i = 0; i < nums.size(); i++) {
bucket[nums[i]]++;
}
int index = 0;
for (int i = 0; i < bucket.size(); i++) {
for (int j = 0; j < bucket[i]; j++) {
nums[index++] = i;
}
}
}
int main() {
vector<int> nums = {5, 3, 2, 8, 6, 4, 1, 9, 0, 7};
int maxVal = *max_element(nums.begin(), nums.end());
bucketSort(nums, maxVal);
for (int i = 0; i < nums.size(); i++) {
cout << nums[i] << " ";
}
return 0;
}
```
这个代码中,我们首先定义了一个桶的数组,大小为最大值加1,然后遍历待排序的数组,将每个元素放入对应的桶中。接着,我们遍历桶数组,将桶中的元素按顺序放回原数组中,最终得到有序的数组。
阅读全文
相关推荐




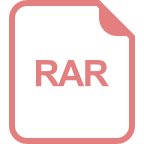










