采用循环左移和循环右移函数控制8个LED灯实现从左向右再从右向左的流水灯代码
时间: 2024-09-13 15:09:09 浏览: 45
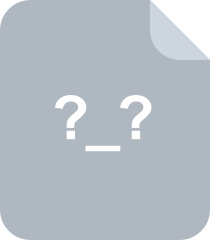
51单片机使用循环左移_crol_(a,b)和循环右移函数_cror_(a,b)实现双向流水灯

要通过循环左移和循环右移函数控制8个LED灯实现流水灯效果,我们可以使用编程语言如C++编写一段代码。这里是一个简单的示例,假设我们有一个数组来表示LED的状态,并且`leds`数组长度为8:
```cpp
#include <iostream>
using namespace std;
// 循环左移
void leftShift(int leds[], int shiftCount) {
for (int i = shiftCount; i < 8; i++) {
leds[i] = leds[i - shiftCount];
}
for (int i = 0; i < shiftCount; i++) {
leds[7 - i] = leds[i];
}
}
// 循环右移
void rightShift(int leds[], int shiftCount) {
for (int i = 0; i < shiftCount; i++) {
leds[i] = leds[i + shiftCount];
}
for (int i = 8 - shiftCount; i < 8; i++) {
leds[i] = leds[i - shiftCount];
}
}
// 更新LED状态
void updateLeds(int leds[]) {
// 这里可以添加你的LED驱动或者其他逻辑
for (int led : leds) {
cout << "LED " << (led == 1 ? "on" : "off") << endl;
}
cout << "\n";
}
int main() {
int leds[8] = {0, 0, 0, 0, 0, 0, 0, 0}; // 初始所有LED关闭
for (int step = 0; ; step++) {
if (step % 2 == 0) { // 每两步做一次循环左移
leftShift(leds, 1); // 左移一位
updateLeds(leds);
} else { // 右移
rightShift(leds, 1);
updateLeds(leds);
}
}
return 0;
}
```
这个代码片段会创建一个无限循环,每两步完成一次左移或右移操作,LED的状态就会呈现出从左向右再从右向左的流水灯效果。
阅读全文
相关推荐
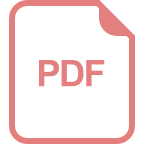
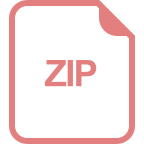
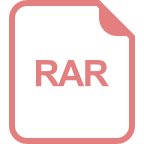

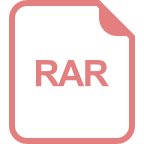
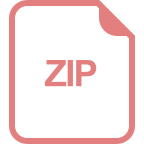
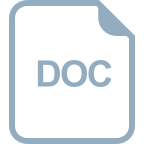
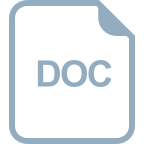









