spring boot 读取文件地址,doc转docx,输出文件流
时间: 2024-01-29 14:04:32 浏览: 273
要在Spring Boot中读取文件地址,并将其转换为docx格式并输出文件流,你可以使用Apache POI库的XWPFDocument类来实现。下面是一个示例代码:
```java
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.springframework.core.io.InputStreamResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import java.io.*;
import java.util.UUID;
@Controller
@RequestMapping("/convert")
public class DocxConversionController {
@GetMapping(value = "/doc-to-docx", produces = MediaType.APPLICATION_OCTET_STREAM_VALUE)
public ResponseEntity<InputStreamResource> convertDocToDocx(@RequestParam String filePath) throws IOException {
// 读取doc文件
FileInputStream inputStream = new FileInputStream(filePath);
XWPFDocument document = new XWPFDocument(inputStream);
// 创建输出流
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
// 写入docx内容到输出流
document.write(outputStream);
outputStream.close();
// 设置响应头
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Disposition", "attachment; filename=output.docx");
// 返回文件流
return ResponseEntity
.ok()
.headers(headers)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(new InputStreamResource(new ByteArrayInputStream(outputStream.toByteArray())));
}
}
```
在上面的示例代码中,我们创建了一个名为`DocxConversionController`的控制器,并使用`convertDocToDocx()`方法来处理转换请求。该方法接收一个名为`filePath`的请求参数,表示要转换的doc文件路径。
我们使用`FileInputStream`读取doc文件,并使用`XWPFDocument`加载文件内容。然后,我们创建一个`ByteArrayOutputStream`作为输出流,并将docx内容写入其中。最后,我们设置响应头,将输出流作为文件流返回给客户端。
请确保在你的Spring Boot应用程序中配置了合适的文件路径,并通过访问`/convert/doc-to-docx?filePath=YOUR_FILE_PATH`来触发转换过程。将`YOUR_FILE_PATH`替换为实际的doc文件路径。返回的响应将是一个`.docx`文件流,可以保存为文件或进行其他处理。
阅读全文
相关推荐
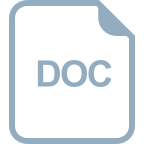
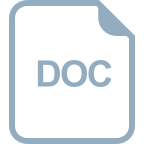
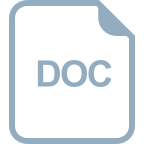


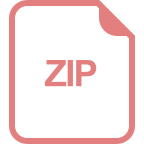

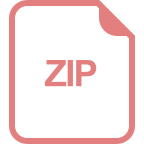
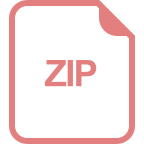
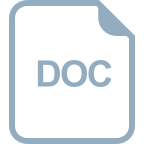








