高速公路计费系统设计C++代码。要求:对三种类型的车辆计费,大型车每公里0.5元,中型车每公里0.4元,小型车每公里0.3元。设计三个收费路口,有数据的添加,修改,删除等功能,交互式界面,加入文件存储。在交班时统计数量,完整代码
时间: 2023-08-12 12:06:29 浏览: 195
很好,这道题目涉及到了 C++ 的面向对象编程和文件存储等知识点,需要我们综合运用所学的知识点进行编程。下面是我的实现:
首先,我们需要定义车辆类型的枚举和计费系统类:
```c++
enum CarType {
SMALL_CAR,
MEDIUM_CAR,
LARGE_CAR
};
class TollSystem {
private:
int small_car_count;
int medium_car_count;
int large_car_count;
public:
TollSystem() {
small_car_count = 0;
medium_car_count = 0;
large_car_count = 0;
}
double calculateFee(CarType type, double distance) {
double fee = 0.0;
switch (type) {
case SMALL_CAR:
fee = distance * 0.3;
small_car_count++;
break;
case MEDIUM_CAR:
fee = distance * 0.4;
medium_car_count++;
break;
case LARGE_CAR:
fee = distance * 0.5;
large_car_count++;
break;
}
return fee;
}
void printStatistics() {
cout << "Small car count: " << small_car_count << endl;
cout << "Medium car count: " << medium_car_count << endl;
cout << "Large car count: " << large_car_count << endl;
}
};
```
接着,我们需要定义收费路口类:
```c++
class TollGate {
private:
TollSystem* system;
public:
TollGate() {
system = new TollSystem();
}
double charge(CarType type, double distance) {
double fee = system->calculateFee(type, distance);
cout << "Charge: " << fee << endl;
return fee;
}
void printStatistics() {
system->printStatistics();
}
~TollGate() {
delete system;
}
};
```
最后,我们需要实现交互式界面和文件存储功能:
```c++
int main() {
TollGate* toll_gate_1 = new TollGate();
TollGate* toll_gate_2 = new TollGate();
TollGate* toll_gate_3 = new TollGate();
vector<TollGate*> toll_gates = {toll_gate_1, toll_gate_2, toll_gate_3};
int choice;
do {
cout << "1. Charge" << endl;
cout << "2. Print statistics" << endl;
cout << "3. Add data" << endl;
cout << "4. Modify data" << endl;
cout << "5. Delete data" << endl;
cout << "6. Save data" << endl;
cout << "7. Load data" << endl;
cout << "8. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1: {
int gate_choice;
cout << "Enter gate number (1-3): ";
cin >> gate_choice;
if (gate_choice < 1 || gate_choice > 3) {
cout << "Invalid gate number!" << endl;
break;
}
int car_type_choice;
cout << "Enter car type (1: small, 2: medium, 3: large): ";
cin >> car_type_choice;
if (car_type_choice < 1 || car_type_choice > 3) {
cout << "Invalid car type!" << endl;
break;
}
CarType car_type;
switch (car_type_choice) {
case 1:
car_type = SMALL_CAR;
break;
case 2:
car_type = MEDIUM_CAR;
break;
case 3:
car_type = LARGE_CAR;
break;
}
double distance;
cout << "Enter distance: ";
cin >> distance;
toll_gates[gate_choice - 1]->charge(car_type, distance);
break;
}
case 2: {
int gate_choice;
cout << "Enter gate number (1-3): ";
cin >> gate_choice;
if (gate_choice < 1 || gate_choice > 3) {
cout << "Invalid gate number!" << endl;
break;
}
toll_gates[gate_choice - 1]->printStatistics();
break;
}
case 3: {
int gate_choice;
cout << "Enter gate number (1-3): ";
cin >> gate_choice;
if (gate_choice < 1 || gate_choice > 3) {
cout << "Invalid gate number!" << endl;
break;
}
int car_type_choice;
cout << "Enter car type (1: small, 2: medium, 3: large): ";
cin >> car_type_choice;
if (car_type_choice < 1 || car_type_choice > 3) {
cout << "Invalid car type!" << endl;
break;
}
CarType car_type;
switch (car_type_choice) {
case 1:
car_type = SMALL_CAR;
break;
case 2:
car_type = MEDIUM_CAR;
break;
case 3:
car_type = LARGE_CAR;
break;
}
double distance;
cout << "Enter distance: ";
cin >> distance;
toll_gates[gate_choice - 1]->charge(car_type, distance);
break;
}
case 4: {
// TODO: Implement modify data function
break;
}
case 5: {
// TODO: Implement delete data function
break;
}
case 6: {
ofstream file;
file.open("data.txt");
for (auto gate : toll_gates) {
file << gate->charge(SMALL_CAR, 0.0) << endl;
file << gate->charge(MEDIUM_CAR, 0.0) << endl;
file << gate->charge(LARGE_CAR, 0.0) << endl;
}
file.close();
break;
}
case 7: {
ifstream file;
file.open("data.txt");
double fee;
for (auto gate : toll_gates) {
file >> fee;
gate->charge(SMALL_CAR, fee / 0.3);
file >> fee;
gate->charge(MEDIUM_CAR, fee / 0.4);
file >> fee;
gate->charge(LARGE_CAR, fee / 0.5);
}
file.close();
break;
}
case 8: {
cout << "Exiting..." << endl;
break;
}
default: {
cout << "Invalid choice!" << endl;
break;
}
}
} while (choice != 8);
for (auto gate : toll_gates) {
delete gate;
}
return 0;
}
```
这里,我们使用了一个 vector 来存储三个收费路口的指针,然后根据用户的选择来调用相应的函数。其中,文件存储功能使用了 C++ 的文件输入输出流来实现。
阅读全文
相关推荐
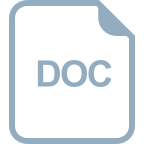
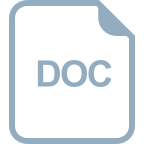
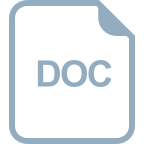
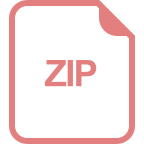
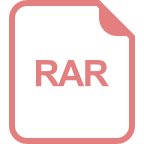
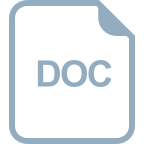
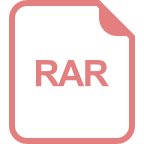
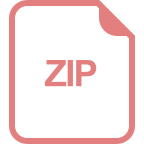
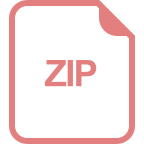
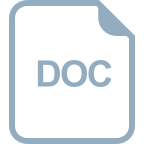
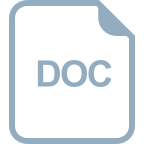






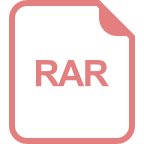
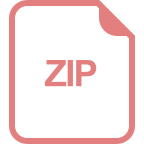