linux tcpdump C++ 代码
时间: 2023-10-21 11:04:54 浏览: 39
以下是一个简单的使用 tcpdump 抓取网络数据包的 C 代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#include <pcap.h>
void print_packet_info(const u_char *packet, struct pcap_pkthdr packet_header);
int main(int argc, char **argv)
{
char *device = NULL;
char error_buffer[PCAP_ERRBUF_SIZE];
pcap_t *handle;
struct bpf_program filter;
char filter_exp[] = "tcp";
bpf_u_int32 subnet_mask, ip;
int num_packets = 10;
if (argc > 1) {
device = argv[1];
} else {
device = pcap_lookupdev(error_buffer);
if (device == NULL) {
fprintf(stderr, "Error finding default device: %s\n", error_buffer);
return 1;
}
}
if (pcap_lookupnet(device, &ip, &subnet_mask, error_buffer) == -1) {
fprintf(stderr, "Error finding device information: %s\n", error_buffer);
return 1;
}
handle = pcap_open_live(device, BUFSIZ, 1, 1000, error_buffer);
if (handle == NULL) {
fprintf(stderr, "Error opening device: %s\n", error_buffer);
return 1;
}
if (pcap_compile(handle, &filter, filter_exp, 0, subnet_mask) == -1) {
fprintf(stderr, "Error compiling filter: %s\n", pcap_geterr(handle));
return 1;
}
if (pcap_setfilter(handle, &filter) == -1) {
fprintf(stderr, "Error setting filter: %s\n", pcap_geterr(handle));
return 1;
}
pcap_loop(handle, num_packets, print_packet_info, NULL);
pcap_close(handle);
return 0;
}
void print_packet_info(const u_char *packet, struct pcap_pkthdr packet_header)
{
struct iphdr *ip_header;
struct tcphdr *tcp_header;
char source_ip[INET_ADDRSTRLEN], dest_ip[INET_ADDRSTRLEN];
u_int source_port, dest_port;
const u_char *payload;
int payload_size;
ip_header = (struct iphdr *)(packet + sizeof(struct ethhdr));
inet_ntop(AF_INET, &(ip_header->saddr), source_ip, INET_ADDRSTRLEN);
inet_ntop(AF_INET, &(ip_header->daddr), dest_ip, INET_ADDRSTRLEN);
tcp_header = (struct tcphdr *)(packet + sizeof(struct ethhdr) + sizeof(struct iphdr));
source_port = ntohs(tcp_header->source);
dest_port = ntohs(tcp_header->dest);
payload = packet + sizeof(struct ethhdr) + sizeof(struct iphdr) + sizeof(struct tcphdr);
payload_size = packet_header.len - (sizeof(struct ethhdr) + sizeof(struct iphdr) + sizeof(struct tcphdr));
printf("Source IP: %s\n", source_ip);
printf("Source Port: %u\n", source_port);
printf("Destination IP: %s\n", dest_ip);
printf("Destination Port: %u\n", dest_port);
printf("Payload Size: %d\n", payload_size);
}
```
这段代码使用 libpcap 库来捕获网络数据包,然后解析出 IP 和 TCP 报文头,打印源 IP、源端口、目标 IP、目标端口和负载大小。使用时可以将命令行参数指定为要抓取的网络设备。如果未指定设备,则将使用默认设备。在此示例中,我们仅仅抓取了 TCP 数据包,可以根据需要更改过滤器表达式。
相关推荐
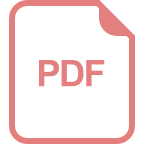













