Java
时间: 2024-02-23 08:03:18 浏览: 14
好的,以下是使用Java实现的程序代码:
```java
import java.util.Scanner;
class Transport {
private int wheelNum;
private double emptyWeight;
Transport(int wheelNum, double emptyWeight) {
this.wheelNum = wheelNum;
this.emptyWeight = emptyWeight;
}
public int getWheelNum() {
return wheelNum;
}
public double getEmptyWeight() {
return emptyWeight;
}
}
class Car extends Transport {
private int passengerNum;
Car(int wheelNum, double emptyWeight, int passengerNum) {
super(wheelNum, emptyWeight);
this.passengerNum = passengerNum;
}
public int getPassengerNum() {
return passengerNum;
}
public double getTotalWeight() {
return getEmptyWeight() + 60 * passengerNum;
}
public String toString() {
return "几轮小车,共载" + passengerNum + "人,总重" + getTotalWeight() + "kg";
}
}
class Truck extends Transport {
private int passengerNum;
private double goodsWeight;
Truck(int wheelNum, double emptyWeight, int passengerNum, double goodsWeight) {
super(wheelNum, emptyWeight);
this.passengerNum = passengerNum;
this.goodsWeight = goodsWeight;
}
public int getPassengerNum() {
return passengerNum;
}
public double getGoodsWeight() {
return goodsWeight;
}
public double getTotalWeight() {
return getEmptyWeight() + 60 * passengerNum + goodsWeight;
}
public String toString() {
return "几轮货车,共载" + passengerNum + "人,载货" + goodsWeight + "kg,总重" + getTotalWeight() + "kg";
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int wheelNum = scanner.nextInt();
double emptyWeight = scanner.nextDouble();
int passengerNum = scanner.nextInt();
double goodsWeight = scanner.nextDouble();
String type = scanner.next();
if (type.equals("小车")) {
Car car = new Car(wheelNum, emptyWeight, passengerNum);
System.out.println(car);
} else if (type.equals("货车")) {
Truck truck = new Truck(wheelNum, emptyWeight, passengerNum, goodsWeight);
System.out.println(truck);
}
scanner.close();
}
}
```
您可以将以上代码保存为Main.java文件并编译运行。
相关推荐
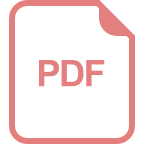
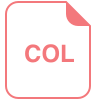
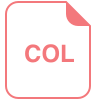
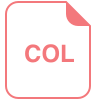
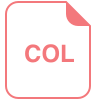
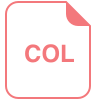










