python numba安装
时间: 2023-11-08 14:03:45 浏览: 87
要安装 Numba,你可以按照以下步骤进行:
1. 确保你已经安装了 Python 解释器和 pip 包管理器。
2. 打开终端或命令提示符,并执行以下命令安装 Numba:
```
pip install numba
```
这将自动从 Python Package Index (PyPI) 下载并安装最新版本的 Numba。
3. 等待安装完成后,你就可以在 Python 代码中导入 Numba 并使用它了。
现在我回答你的
相关问题
python Numba
Numba is a just-in-time (JIT) compiler for Python that translates Python code into optimized machine code at runtime. It is specifically designed to speed up the execution of numerical computations and works well with NumPy arrays and functions.
By using Numba, you can achieve significant performance improvements in Python code without having to rewrite it in a lower-level language like C or Fortran. Numba allows you to write your code in pure Python and then decorate the functions or methods that you want to accelerate with the `@jit` decorator.
Here's an example of how to use Numba to accelerate a Python function:
```python
import numba as nb
@nb.jit
def my_function(x, y):
z = x + y
return z
result = my_function(3, 4)
print(result)
```
In this example, the `@jit` decorator tells Numba to compile the `my_function` function. Numba then generates optimized machine code for the function, resulting in faster execution compared to regular Python code.
It's important to note that not all types of Python code can be accelerated with Numba. It works best with numerical computations that involve arrays, loops, and mathematical operations. Additionally, Numba supports parallel computation on multiple CPU cores and can also generate code for GPUs.
I hope this answers your question! Let me know if you have any more.
python numba prange
Python Numba是一个用于加速Python代码的开源库。它通过即时编译技术将Python代码转换为机器码,从而提供了比原生Python更高的执行速度。
Numba中的prange函数是一个并行循环函数,用于在循环中实现并行计算。它类似于Python内置的range函数,但可以在循环中使用并行计算来提高性能。prange函数可以与Numba的jit装饰器一起使用,以便将循环中的代码编译为机器码并进行并行执行。
使用prange函数的示例代码如下:
```python
from numba import njit, prange
@njit(parallel=True)
def parallel_sum(arr):
total = 0
for i in prange(len(arr)):
total += arr[i]
return total
arr = [1, 2, 3, 4, 5]
result = parallel_sum(arr)
print(result)
```
在上面的示例中,我们定义了一个名为parallel_sum的函数,使用了prange函数来实现并行求和。通过将parallel=True传递给njit装饰器,我们告诉Numba编译器将循环代码转换为并行执行的机器码。然后,我们调用parallel_sum函数,并传递一个包含整数的列表作为参数。最后,我们打印出计算结果。
相关推荐
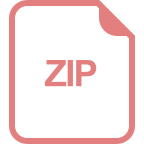












