用C语言定义函数:按学号由小到大,建立有序的链表。逆序输入n个学生信息,学号大的先输入,建立带头结点的单链表。
时间: 2024-04-13 12:29:56 浏览: 12
你可以使用以下代码来定义C语言函数,按照学号由小到大的顺序建立有序的链表,逆序输入n个学生信息:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct Student {
int id;
char name[50];
struct Student* next;
} Student;
// 创建新节点
Student* createNode(int id, char name[]) {
Student* newNode = (Student*)malloc(sizeof(Student));
newNode->id = id;
strcpy(newNode->name, name);
newNode->next = NULL;
return newNode;
}
// 按学号由小到大插入节点
void insertNode(Student** head, int id, char name[]) {
Student* newNode = createNode(id, name);
// 如果链表为空,直接将新节点作为头节点
if (*head == NULL || (*head)->id >= id) {
newNode->next = *head;
*head = newNode;
} else {
Student* current = *head;
// 寻找合适的插入位置
while (current->next != NULL && current->next->id < id) {
current = current->next;
}
// 插入新节点
newNode->next = current->next;
current->next = newNode;
}
}
// 打印链表
void printList(Student* head) {
Student* current = head;
while (current != NULL) {
printf("学号:%d\t姓名:%s\n", current->id, current->name);
current = current->next;
}
}
int main() {
int n, id;
char name[50];
Student* head = NULL; // 初始化链表为空
printf("请输入学生人数:");
scanf("%d", &n);
printf("请逆序输入学生信息(学号 姓名):\n");
for (int i = 0; i < n; i++) {
scanf("%d %s", &id, name);
insertNode(&head, id, name);
}
printf("建立的有序链表如下:\n");
printList(head);
return 0;
}
```
在这段代码中,我们首先定义了学生结构体,包含学号和姓名。然后,我们实现了创建新节点的函数`createNode`,该函数根据传入的学号和姓名创建新节点。接着,我们实现了按学号由小到大插入节点的函数`insertNode`,该函数会根据传入的学号和姓名将新节点插入到合适的位置。最后,我们通过在`main`函数中读取输入的学生信息,并调用`insertNode`函数来建立有序的链表。最后,我们使用`printList`函数打印整个链表。
这样,你就可以按照学号由小到大的顺序建立有序的链表了。
相关推荐
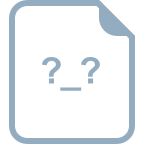
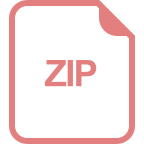
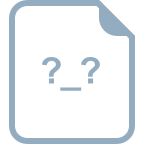
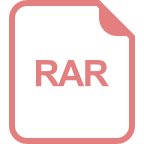
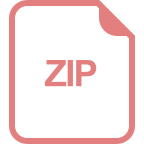
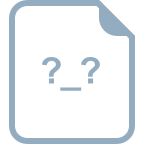
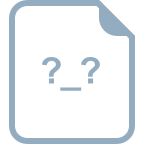
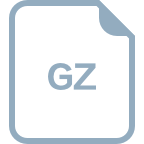
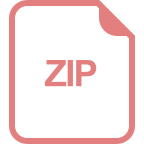
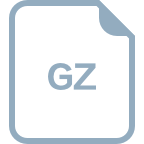
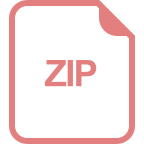