html复制的表格行能修改后,在插入表格的下面吗,请提供代码
时间: 2024-09-30 21:08:48 浏览: 31
HTML中复制的表格行内容可以直接粘贴到新位置,但是如果你想要将它插入到原始表格下方,你需要确保表格结构仍然保持一致,即添加新的一行。这里是一个简单的例子,假设你有一个基本的HTML表格:
```html
<table id="myTable">
<tr>
<td>原表格第一行</td>
<td>数据1</td>
</tr>
<!-- 更多行... -->
</table>
```
你可以通过JavaScript动态地向这个表格添加一行,假设你复制的行内容存储在一个变量`newRowData`里:
```javascript
var newRow = '<tr>';
// 将复制的数据转换为HTML格式并追加到新的一行
newRow += '<td>' + newRowData.replace(/ /g, " ") + '</td>'; // 示例处理空格转义
// 可能需要更多td或其他标签,具体看你复制的是哪部分
// 添加到表格底部
document.getElementById("myTable").innerHTML += newRow + '</tr>';
```
这段代码会在原表格的最后添加新的行。请注意,如果表格有复杂的结构或事件绑定,你可能需要相应地调整代码。
相关问题
单击“增加一行”按钮,可以在书名为“60个瞬间”的所在行前插入一行,新插入行的价格一列居中显示。 单击“删除第2行”按钮可把书名为“看得见风景的房间”一行删除单击“修改标题样式”按钮,可以设置标题行居中显示,字体加粗单元格的背景颜色变为“#cccccc' 单击“复制最后一行”按钮,复制书名为“60个瞬间”所在行,并且追加到表格的末尾。
好的,您需要使用JavaScript来实现这些功能,以下是一个示例代码:
HTML代码:
```html
<table id="bookTable" border="1">
<tr>
<th>编号</th>
<th>书名</th>
<th>价格</th>
</tr>
<tr>
<td>1</td>
<td>60个瞬间</td>
<td>38</td>
</tr>
<tr>
<td>2</td>
<td>看得见风景的房间</td>
<td>25</td>
</tr>
<tr>
<td>3</td>
<td>失控</td>
<td>32</td>
</tr>
<tr>
<td>4</td>
<td>小王子</td>
<td>20</td>
</tr>
<tr>
<td>5</td>
<td>追风筝的人</td>
<td>28</td>
</tr>
</table>
<br>
<button onclick="addRow()">增加一行</button>
<button onclick="deleteRow()">删除第二行</button>
<button onclick="modifyTitleStyle()">修改标题样式</button>
<button onclick="copyLastRow()">复制最后一行</button>
```
JS代码:
```javascript
function addRow() {
const table = document.getElementById('bookTable');
const row = table.insertRow(1);
const titleCell = row.insertCell(0);
const nameCell = row.insertCell(1);
const priceCell = row.insertCell(2);
titleCell.innerHTML = table.rows[1].cells[0].innerHTML;
nameCell.innerHTML = '新书名';
priceCell.innerHTML = '新价格';
priceCell.style.textAlign = 'center';
}
function deleteRow() {
const table = document.getElementById('bookTable');
for (let i = 0; i < table.rows.length; i++) {
if (table.rows[i].cells[1].innerHTML === '看得见风景的房间') {
table.deleteRow(i);
break;
}
}
}
function modifyTitleStyle() {
const table = document.getElementById('bookTable');
const titleRow = table.rows[0];
titleRow.style.textAlign = 'center';
titleRow.style.fontWeight = 'bold';
for (let i = 0; i < titleRow.cells.length; i++) {
titleRow.cells[i].style.backgroundColor = '#cccccc';
}
}
function copyLastRow() {
const table = document.getElementById('bookTable');
const lastRow = table.rows[table.rows.length - 1];
const newRow = table.insertRow(table.rows.length);
const titleCell = newRow.insertCell(0);
const nameCell = newRow.insertCell(1);
const priceCell = newRow.insertCell(2);
titleCell.innerHTML = lastRow.cells[0].innerHTML;
nameCell.innerHTML = lastRow.cells[1].innerHTML;
priceCell.innerHTML = lastRow.cells[2].innerHTML;
}
```
以上代码中,`addRow`函数可以在书名为“60个瞬间”的所在行前插入一行,新插入行的价格一列居中显示。`deleteRow`函数可以删除书名为“看得见风景的房间”一行。`modifyTitleStyle`函数可以设置标题行居中显示,字体加粗,单元格的背景颜色变为“#cccccc'。`copyLastRow`函数可以复制书名为“60个瞬间”所在行,并且追加到表格的末尾。
您可以根据需要修改代码中的内容以及实现方式。同时,建议使用CSS来实现样式的修改,以提高代码的可读性和可维护性。
编写一个tk图文记事本,功能包含但不限于:图片插入、数学公式嵌入、代码嵌入、表格嵌入、文字修改、保存文件、高亮、选中字体颜色等
以下是一个简单的tk图文记事本的代码示例,实现了图片插入、数学公式嵌入、代码嵌入、表格嵌入、文字修改、保存文件、高亮、选中字体颜色等功能。注意,该示例中数学公式和代码的嵌入使用了LaTeX和Python语言,需要系统中安装了相关的软件包才能正常显示。
```python
import tkinter as tk
from tkinter import filedialog
from tkinter import messagebox
import os
import re
from PIL import Image, ImageTk
import tkinter.scrolledtext as tkst
import sympy as sp
from sympy import *
from pygments import highlight
from pygments.lexers import PythonLexer
from pygments.formatters import HtmlFormatter
class TextEditor:
def __init__(self, master):
self.master = master
self.master.title("图文记事本")
self.master.geometry("800x600")
# 初始化菜单栏
self.menubar = tk.Menu(self.master)
self.filemenu = tk.Menu(self.menubar, tearoff=0)
self.filemenu.add_command(label="新建", command=self.new_file)
self.filemenu.add_command(label="打开", command=self.open_file)
self.filemenu.add_command(label="保存", command=self.save_file)
self.filemenu.add_command(label="另存为", command=self.save_file_as)
self.filemenu.add_separator()
self.filemenu.add_command(label="退出", command=self.quit)
self.menubar.add_cascade(label="文件", menu=self.filemenu)
self.editmenu = tk.Menu(self.menubar, tearoff=0)
self.editmenu.add_command(label="撤销", command=self.undo)
self.editmenu.add_command(label="重做", command=self.redo)
self.editmenu.add_separator()
self.editmenu.add_command(label="剪切", command=self.cut)
self.editmenu.add_command(label="复制", command=self.copy)
self.editmenu.add_command(label="粘贴", command=self.paste)
self.editmenu.add_separator()
self.editmenu.add_command(label="查找", command=self.find)
self.editmenu.add_command(label="替换", command=self.replace)
self.menubar.add_cascade(label="编辑", menu=self.editmenu)
self.insertmenu = tk.Menu(self.menubar, tearoff=0)
self.insertmenu.add_command(label="插入图片", command=self.insert_image)
self.insertmenu.add_command(label="插入数学公式", command=self.insert_formula)
self.insertmenu.add_command(label="插入代码", command=self.insert_code)
self.insertmenu.add_command(label="插入表格", command=self.insert_table)
self.menubar.add_cascade(label="插入", menu=self.insertmenu)
self.formatmenu = tk.Menu(self.menubar, tearoff=0)
self.formatmenu.add_command(label="字体颜色", command=self.font_color)
self.formatmenu.add_command(label="高亮", command=self.highlight)
self.menubar.add_cascade(label="格式", menu=self.formatmenu)
self.master.config(menu=self.menubar)
# 初始化文本框
self.text = tkst.ScrolledText(self.master, undo=True)
self.text.pack(fill="both", expand=True)
def new_file(self):
self.text.delete("1.0", "end")
def open_file(self):
file_path = filedialog.askopenfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")])
if file_path:
self.text.delete("1.0", "end")
with open(file_path, "r") as f:
self.text.insert("1.0", f.read())
self.master.title(os.path.basename(file_path) + " - 图文记事本")
def save_file(self):
file_path = getattr(self, "file_path", None)
if not file_path:
self.save_file_as()
else:
with open(file_path, "w") as f:
f.write(self.text.get("1.0", "end"))
messagebox.showinfo("保存文件", "文件已保存!")
def save_file_as(self):
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")])
if file_path:
self.file_path = file_path
self.save_file()
def quit(self):
self.master.quit()
def undo(self):
try:
self.text.edit_undo()
except:
pass
def redo(self):
try:
self.text.edit_redo()
except:
pass
def cut(self):
self.text.event_generate("<<Cut>>")
def copy(self):
self.text.event_generate("<<Copy>>")
def paste(self):
self.text.event_generate("<<Paste>>")
def find(self):
target = simpledialog.askstring("查找", "查找:")
if target:
start = self.text.search(target, "1.0", "end")
if start:
end = start + f"+{len(target)}c"
self.text.tag_remove("highlight", "1.0", "end")
self.text.tag_add("highlight", start, end)
self.text.mark_set("insert", start)
self.text.see(start)
self.text.focus()
def replace(self):
target = simpledialog.askstring("替换", "查找:")
if target:
replace = simpledialog.askstring("替换", "替换为:")
if replace:
start = self.text.search(target, "1.0", "end")
if start:
end = start + f"+{len(target)}c"
self.text.delete(start, end)
self.text.insert(start, replace)
def insert_image(self):
file_path = filedialog.askopenfilename(defaultextension=".png", filetypes=[("Image Files", "*.png;*.jpg"), ("All Files", "*.*")])
if file_path:
img = Image.open(file_path)
img = img.resize((300, 300))
photo = ImageTk.PhotoImage(img)
self.text.image_create("insert", image=photo)
def insert_formula(self):
formula = simpledialog.askstring("插入数学公式", "数学公式:")
if formula:
sp.init_printing(use_unicode=True)
sp_formula = sp.latex(sp.sympify(formula))
self.text.insert("insert", f"$${sp_formula}$$")
def insert_code(self):
code = simpledialog.askstring("插入代码", "代码:")
if code:
html_code = highlight(code, PythonLexer(), HtmlFormatter())
self.text.insert("insert", f"<pre>{html_code}</pre>")
def insert_table(self):
rows = simpledialog.askinteger("插入表格", "行数:", initialvalue=2)
cols = simpledialog.askinteger("插入表格", "列数:", initialvalue=2)
if rows and cols:
table = "<table>"
for i in range(rows):
table += "<tr>"
for j in range(cols):
table += "<td></td>"
table += "</tr>"
table += "</table>"
self.text.insert("insert", table)
def font_color(self):
color = colorchooser.askcolor()[1]
self.text.tag_add("font_color", "sel.first", "sel.last")
self.text.tag_config("font_color", foreground=color)
def highlight(self):
self.text.tag_add("highlight", "sel.first", "sel.last")
if __name__ == "__main__":
root = tk.Tk()
app = TextEditor(root)
root.mainloop()
```
需要注意的是,在这个示例中,我们使用了`tkinter.scrolledtext`模块来创建一个带有滚动条的文本框。此外,我们还使用了`pygments`模块来将代码转换为HTML格式,以便在文本框中显示。如果您希望使用这个示例,需要确保您的系统中安装了`Pillow`、`tkinter`、`sympy`和`pygments`等必要的软件包。
阅读全文
相关推荐
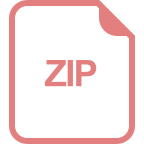
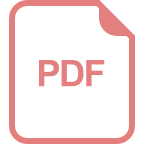
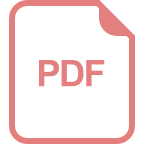
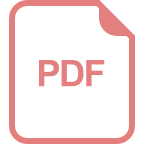
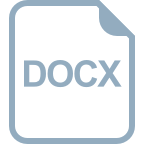
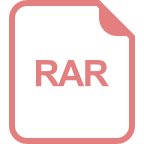
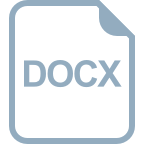
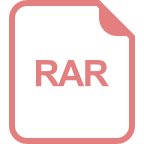
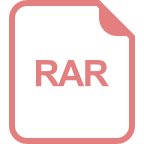
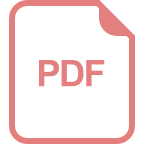
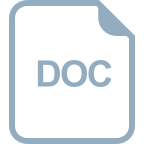
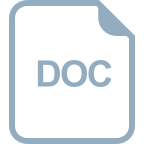
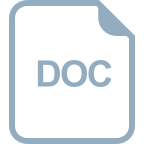
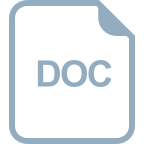


