用java实现C++中得库函数std::arg(std::complex)
时间: 2023-07-08 19:40:19 浏览: 250
在Java中,可以使用 `java.util.function.Function` 接口来实现类似于 C++ 中的 `std::arg(std::complex)` 函数。
首先,我们可以定义一个 `Complex` 类表示复数,包含实部和虚部:
```java
class Complex {
private double real;
private double imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public double getReal() {
return this.real;
}
public double getImaginary() {
return this.imaginary;
}
}
```
然后,我们可以定义一个 `arg` 函数,该函数接受一个 `Complex` 对象作为参数,并返回该复数的幅角(弧度制):
```java
import java.util.function.Function;
public class Main {
public static void main(String[] args) {
// 创建一个复数对象
Complex z = new Complex(1.0, 1.0);
// 调用 arg 函数获取幅角
double arg = arg(z);
// 输出结果
System.out.println(arg);
}
public static double arg(Complex z) {
// 使用 Function 接口计算幅角
Function<Complex, Double> argFunc = (c) -> Math.atan2(c.getImaginary(), c.getReal());
// 调用 apply 方法获取结果
return argFunc.apply(z);
}
}
```
在 `arg` 函数中,我们定义了一个 `Function` 对象 `argFunc`,该对象接受一个 `Complex` 对象作为参数,并使用 `Math.atan2()` 方法计算幅角。然后,我们调用 `apply()` 方法,将复数对象传递给 `argFunc`,得到幅角的结果。
注意,`Math.atan2()` 方法返回的是弧度制的幅角,与 C++ 中的 `std::arg()` 函数一致。如果需要得到角度制的幅角,可以将结果乘以 `180.0/Math.PI`。
阅读全文
相关推荐
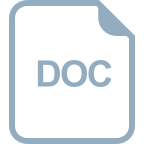
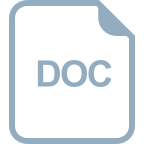
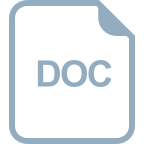
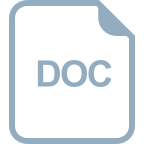
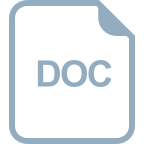
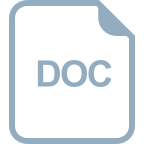
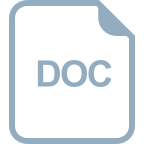
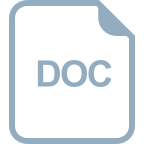







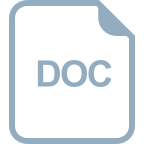