c语言调用RSA公钥加密
时间: 2023-08-24 15:09:50 浏览: 56
以下是一个简单的示例代码,演示如何使用openssl库调用RSA公钥加密函数:
```c
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
int main()
{
// 加载公钥
FILE* fp = fopen("public.key", "rb");
RSA* rsa = PEM_read_RSA_PUBKEY(fp, NULL, NULL, NULL);
fclose(fp);
if (rsa == NULL) {
fprintf(stderr, "Error loading public key: %s\n", ERR_error_string(ERR_get_error(), NULL));
return 1;
}
// 待加密的数据
const char* plain_text = "Hello, RSA!";
int plain_text_len = strlen(plain_text);
// 分配内存存储加密后的数据
int max_cipher_text_len = RSA_size(rsa);
unsigned char* cipher_text = (unsigned char*)malloc(max_cipher_text_len);
if (cipher_text == NULL) {
fprintf(stderr, "Error allocating memory for cipher text.\n");
RSA_free(rsa);
return 1;
}
// 加密
int cipher_text_len = RSA_public_encrypt(plain_text_len, (unsigned char*)plain_text, cipher_text, rsa, RSA_PKCS1_PADDING);
if (cipher_text_len == -1) {
fprintf(stderr, "Error encrypting data: %s\n", ERR_error_string(ERR_get_error(), NULL));
RSA_free(rsa);
free(cipher_text);
return 1;
}
// 输出加密后的数据
printf("Encrypted data (hex):\n");
for (int i = 0; i < cipher_text_len; i++) {
printf("%02x", cipher_text[i]);
}
printf("\n");
// 释放资源
RSA_free(rsa);
free(cipher_text);
return 0;
}
```
在以上示例代码中,我们首先通过`PEM_read_RSA_PUBKEY`函数加载公钥文件,然后分配内存存储加密结果,最后调用`RSA_public_encrypt`函数进行加密。注意,我们使用了PKCS#1 v1.5填充方案(`RSA_PKCS1_PADDING`)进行加密。加密后的结果以十六进制形式输出。
需要注意的是,以上示例代码仅作为演示用途,实际使用时需要进行错误处理和内存管理。同时,我们也应该注意保护私钥,避免泄露。
相关推荐
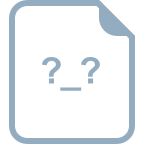
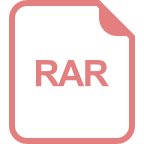














