解释filePath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' for i in os.listdir(filePath): outPath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' + str(i) img = cv.imread("./deblur/."+i) img_ = motion_blur1(img) cv.imwrite(outPath,img_)
时间: 2023-07-21 20:54:08 浏览: 221
这段代码主要是对指定路径下的图像进行运动模糊处理,并将结果保存到指定路径中。解释如下:
- 第一行定义了一个字符串变量 `filePath`,其值为待处理图像所在的文件夹路径,即 `D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.`
- 第二行使用 `os.listdir()` 函数获取该文件夹下所有文件的文件名,并通过 `for` 循环遍历每个文件名(用变量 `i` 代表)。
- 第三行定义了一个字符串变量 `outPath`,其值为处理后的图像保存的路径,即 `D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.` 加上当前遍历到的文件名 `i`。
- 第四行使用 `cv.imread()` 函数读取待处理的图像文件,其路径为 `./deblur/.` 加上当前遍历到的文件名 `i`。
- 第五行调用 `motion_blur1()` 函数对读入的图像进行运动模糊处理,并将处理结果保存到 `img_` 变量中。
- 第六行使用 `cv.imwrite()` 函数将处理结果保存到指定路径 `outPath` 中。
总体来说,这段代码是对指定路径下的所有图像进行运动模糊处理,并将处理结果保存到指定路径中。
相关问题
def motion_blur1(image, degree=10, angle=45): image = np.array(image) # 这里生成任意角度的运动模糊kernel的矩阵, degree越大,模糊程度越高 M = cv.getRotationMatrix2D((degree / 2, degree / 2), angle, 1) motion_blur_kernel = np.diag(np.ones(degree)) motion_blur_kernel = cv.warpAffine(motion_blur_kernel, M, (degree, degree)) motion_blur_kernel = motion_blur_kernel / degree blurred = cv.filter2D(image, -1, motion_blur_kernel) # convert to uint8 cv.normalize(blurred, blurred, 0, 255, cv.NORM_MINMAX) blurred = np.array(blurred, dtype=np.uint8) return blurred filePath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur' for i in os.listdir(filePath): outPath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' + str(i) img = cv.imread("./deblur/"+i) img_ = motion_blur1(img) cv.imwrite(outPath,img_),上述代码出现问题:cv2.error: OpenCV(4.7.0) :-1: error: (-5:Bad argument) in function 'filter2D' > Overload resolution failed: > - src data type = 17 is not supported > - Expected Ptrcv::UMat for argument 'src'
在你的代码中,img变量的类型是一个普通的numpy数组,它的数据类型为uint8。为了解决这个问题,你可以将img变量转换为OpenCV的UMat类型,这是OpenCV中的一种特殊类型,它可以更好地与OpenCV的函数一起使用。
你可以使用cv2.UMat()函数将numpy数组转换为UMat类型。修改代码如下:
```
filePath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur'
for i in os.listdir(filePath):
outPath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' + str(i)
img = cv.imread("./deblur/"+i)
img = cv.UMat(img) # 将numpy数组转换为UMat类型
img_ = motion_blur1(img)
cv.imwrite(outPath,img_)
```
这样应该就能解决上述错误了。如果还有问题,请提供更多的代码和错误信息,以便我们更好地帮助你解决问题。
coding: utf-8 import cv2 import numpy as np import cv2 as cv import os def motion_blur1(image, degree=10, angle=45): image = np.array(image) # 这里生成任意角度的运动模糊kernel的矩阵, degree越大,模糊程度越高 M = cv.getRotationMatrix2D((degree / 2, degree / 2), angle, 1) motion_blur_kernel = np.diag(np.ones(degree)) motion_blur_kernel = cv.warpAffine(motion_blur_kernel, M, (degree, degree)) motion_blur_kernel = motion_blur_kernel / degree blurred = cv.filter2D(image, -1, motion_blur_kernel) # convert to uint8 cv.normalize(blurred, blurred, 0, 255, cv.NORM_MINMAX) blurred = np.array(blurred, dtype=np.uint8) return blurred filePath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur' for i in os.listdir(filePath): outPath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' + str(i) img = cv.imread("./deblur/"+i) img = cv.UMat(img) # 将numpy数组转换为UMat类型 img_ = motion_blur1(img) cv.imwrite(outPath,img_),上述代码出现问题:cv2.error: OpenCV(4.7.0) :-1: error: (-5:Bad argument) in function 'filter2D' > Overload resolution failed: > - src data type = 17 is not supported > - Expected Ptrcv::UMat for argument 'src'
这个错误是由于filter2D函数的输入类型不匹配导致的。在这里,您正在使用UMat类型的图像,但是filter2D函数不支持UMat类型的图像。您可以尝试将UMat类型的图像转换为numpy数组类型的图像,然后再进行模糊处理。这里是修改后的代码:
```python
import cv2
import numpy as np
import os
def motion_blur1(image, degree=10, angle=45):
image = np.array(image)
# 生成任意角度的运动模糊kernel的矩阵, degree越大,模糊程度越高
M = cv2.getRotationMatrix2D((degree / 2, degree / 2), angle, 1)
motion_blur_kernel = np.diag(np.ones(degree))
motion_blur_kernel = cv2.warpAffine(motion_blur_kernel, M, (degree, degree))
motion_blur_kernel = motion_blur_kernel / degree
# 将UMat类型的图像转换为numpy数组类型的图像
blurred = cv2.filter2D(image.get(), -1, motion_blur_kernel)
# convert to uint8
cv2.normalize(blurred, blurred, 0, 255, cv2.NORM_MINMAX)
blurred = np.array(blurred, dtype=np.uint8)
# 将numpy数组类型的图像转换为UMat类型的图像
blurred = cv2.UMat(blurred)
return blurred
filePath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur'
for i in os.listdir(filePath):
outPath = r'D:\Users\Administrator\PycharmProjects\pythonProject\paper_need\blur.' + str(i)
img = cv2.imread("./deblur/"+i)
img = cv2.UMat(img)
img_ = motion_blur1(img)
cv2.imwrite(outPath,img_.get())
```
这里将UMat类型的图像转换为numpy数组类型的图像,然后进行模糊处理,最后再将numpy数组类型的图像转换为UMat类型的图像。这样就可以解决这个问题了。
阅读全文
相关推荐
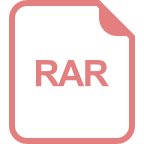
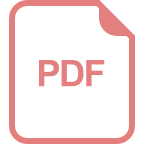
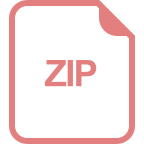

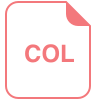
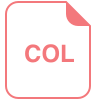
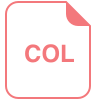
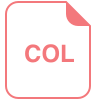
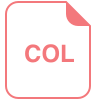
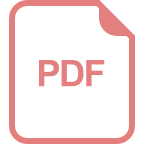
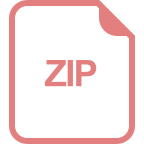
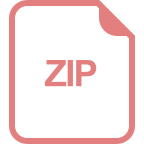
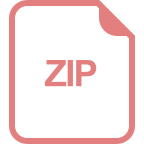