用c++语言,开头添加using namespace std不用sort函数请用户输入4首歌名,歌名存入一字符指针数组,然后分别按原序、字母序和字母逆序(从Z到A)显示这些歌名。
时间: 2024-10-21 11:11:58 浏览: 14
在C++中,你可以使用`std::cin`从用户那里获取输入,存储在字符指针数组中,然后使用自定义的比较函数对歌名进行排序。这里是一个简单的示例,展示了如何按照要求操作:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义比较函数用于原序、字母顺序和逆序排序
bool compareAlphabetically(const char* a, const char* b) {
return strcmp(a, b) < 0;
}
bool compareReverseAlphabetically(const char* a, const char* b) {
return strcmp(b, a) < 0;
}
int main() {
const int numSongs = 4;
char songNames[numSongs][50]; // 假设每个歌名最多为50个字符
cout << "请输入4首歌名:" << endl;
for (int i = 0; i < numSongs; ++i) {
cin >> songNames[i];
}
// 原序显示
cout << "原始顺序:";
for (int i = 0; i < numSongs; ++i) {
cout << songNames[i] << " ";
}
cout << endl;
// 按字母顺序显示
sort(songNames, songNames + numSongs, compareAlphabetically);
cout << "字母顺序:";
for (int i = 0; i < numSongs; ++i) {
cout << songNames[i] << " ";
}
cout << endl;
// 按字母逆序显示
sort(songNames, songNames + numSongs, compareReverseAlphabetically);
cout << "字母逆序:";
for (int i = 0; i < numSongs; ++i) {
cout << songNames[i] << " ";
}
cout << endl;
return 0;
}
```
在这个例子中,我们首先定义了两个比较函数`compareAlphabetically`和`compareReverseAlphabetically`,它们分别根据字典顺序和反字典顺序来判断歌名的顺序。接着,在`main`函数中,我们获取用户输入的歌名并存储在数组中,然后调用`std::sort`函数三次,每次传入不同的比较函数。
阅读全文
相关推荐
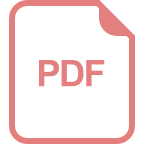
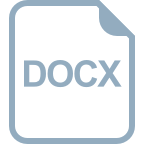
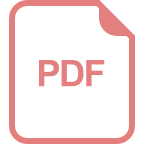



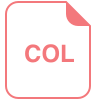
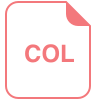








