舞伴问题(队列) 男士: (大写字母表示) 女士:(小写字母表示 第一步:定义两个队列Man,Women; InitQueue(M), InitQueue(W) 第二步:从键盘输入一串字符(大小写混合,#表示结束) 对每一个个字符进行判断,大写字母就入队(Man),小写字母入(Women),循环输入字符,判断后EnQueue(M,x),判断后EnQueue(W,x) 第三步:舞伴配对:Man队列出队一个,对应的vomen队人列也要出队一个(循环执行,结束条件:其中一个队 列变空) DeQueue(M, x), DeQueue(W, x), 结果工 ManB列和women队人列都空,提示”success” 结果2:Man队列为空, • women队列不空,提示:“sorry madam,you should wait' QueueEmpty(M) 结果1:women队列为空,Man队列不空,提示: "sorry sir,you should wait!"
时间: 2024-02-01 10:15:47 浏览: 91
以下是代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#define MAXSIZE 100
typedef struct {
char data[MAXSIZE];
int front;
int rear;
} Queue;
void InitQueue(Queue *Q) {
Q->front = Q->rear = 0;
}
bool IsEmpty(Queue Q) {
return Q.front == Q.rear;
}
bool IsFull(Queue Q) {
return (Q.rear + 1) % MAXSIZE == Q.front;
}
bool EnQueue(Queue *Q, char x) {
if (IsFull(*Q)) {
return false;
}
Q->data[Q->rear] = x;
Q->rear = (Q->rear + 1) % MAXSIZE;
return true;
}
bool DeQueue(Queue *Q, char *x) {
if (IsEmpty(*Q)) {
return false;
}
*x = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return true;
}
int main() {
Queue Man, Women;
InitQueue(&Man);
InitQueue(&Women);
char c;
printf("Please input a string (including uppercase and lowercase letters, end with #):\n");
while ((c = getchar()) != '#') {
if (isupper(c)) {
EnQueue(&Man, c);
} else if (islower(c)) {
EnQueue(&Women, c);
}
}
while (!IsEmpty(Man) && !IsEmpty(Women)) {
char man, woman;
DeQueue(&Man, &man);
DeQueue(&Women, &woman);
printf("%c-%c\n", man, woman);
}
if (!IsEmpty(Man)) {
printf("sorry sir, you should wait!\n");
} else if (!IsEmpty(Women)) {
printf("sorry madam, you should wait!\n");
} else {
printf("success!\n");
}
return 0;
}
```
代码思路:
首先定义两个队列 Man 和 Women,分别用来存储男士和女士的信息。然后从键盘输入一个字符串,对每一个字符进行判断,如果是大写字母,则将其入队到 Man 队列,如果是小写字母,则将其入队到 Women 队列。接下来,循环执行出队操作,每次从 Man 和 Women 队列中各取出一个元素,表示男士和女士配对,输出他们的信息,并继续循环,直到其中一个队列为空。最后,根据 Man 和 Women 队列是否为空,输出相应的提示信息。
阅读全文
相关推荐
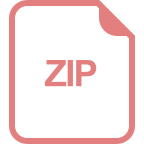

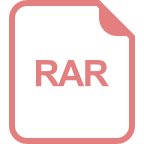
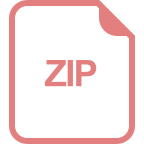
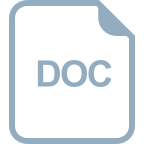











