用VHDL实现LED灯从右到左波动点亮
时间: 2024-05-11 08:18:58 浏览: 12
首先,我们需要确定硬件平台,选择一个FPGA开发板,例如Xilinx Spartan-6 FPGA开发板。
然后,我们需要编写VHDL代码来实现LED灯从右到左波动点亮。
以下是一个可能的实现:
```vhdl
--定义时钟频率
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity led_wave is
port (
clk : in std_logic; --时钟输入
leds : out std_logic_vector(7 downto 0) --LED输出
);
end entity;
architecture Behavioral of led_wave is
constant CLK_FREQ : integer := 50000000; --时钟频率
constant WAVE_FREQ : integer := 2; --波动频率
constant WAVE_WIDTH : integer := 8; --波动宽度
signal counter : unsigned(31 downto 0); --计数器
signal wave_pos : integer range 0 to WAVE_WIDTH-1; --波动位置
begin
--时钟分频
process(clk)
begin
if rising_edge(clk) then
counter <= counter + 1;
end if;
end process;
--波动点亮
process(counter)
begin
if counter'event and counter = CLK_FREQ / WAVE_FREQ then
counter <= (others => '0');
wave_pos <= (wave_pos + 1) mod WAVE_WIDTH;
leds <= std_logic_vector(shift_right(unsigned(to_unsigned(255, 8)), wave_pos));
end if;
end process;
end architecture;
```
该代码定义了一个名为`led_wave`的实体,它具有一个时钟输入`clk`和一个8位LED输出`leds`。它使用了一个计数器和一个波动位置信号来控制LED的波动点亮。
在时钟分频进程中,计数器每个时钟周期加一。在波动点亮进程中,当计数器达到指定的计数值时,波动位置加一,并将LED输出设置为一个8位向右移动了波动位置的255值。
为了将代码加载到FPGA开发板中,我们需要使用Xilinx ISE工具链,将代码综合、映射、布局和路由,并生成一个比特流文件,然后将该文件下载到FPGA开发板中。
相关推荐
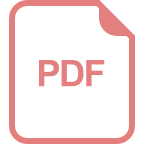
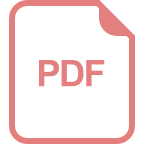
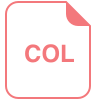
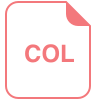
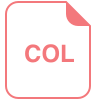
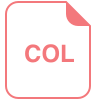
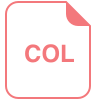









