python如何读取config.properties里面的配置项
时间: 2024-02-03 09:14:46 浏览: 67
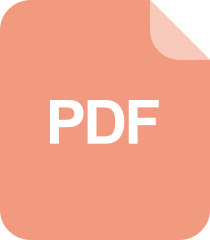
Python实现读取Properties配置文件的方法
可以使用Python的ConfigParser模块来读取config.properties文件中的配置项。以下是一个示例代码:
```python
import configparser
config = configparser.ConfigParser()
config.read('config.properties')
# 读取单个配置项
value = config.get('section_name', 'property_name')
# 读取整个section的配置项
section = config['section_name']
```
需要注意的是,ConfigParser模块只能读取以“键-值”形式存储的配置文件(如config.properties),如果配置文件中有其他格式,需要使用其他模块进行解析。
阅读全文
相关推荐


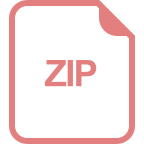
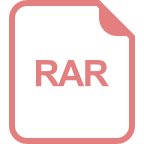
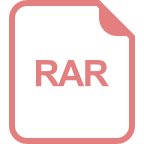
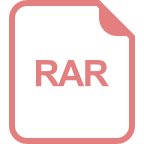
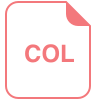
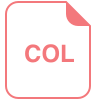
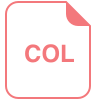
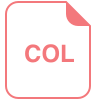
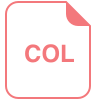
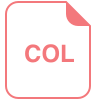
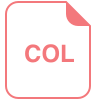
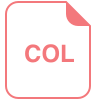
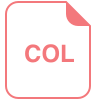
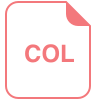