用vue写一段代码用户可以自建不限数量的单词书。 在创建单词书时,需要用户自己输入单词以及单词的词性、中文释义。 用户创建完单词书后,仍然可以对已创建的单词书进行局部的增、删、改。
时间: 2024-06-04 13:11:31 浏览: 27
以下是基于Vue的代码示例,用于实现用户自建不限数量的单词书:
<template>
<div>
<h2>创建/编辑单词书</h2>
<form>
<div class="form-control">
<label>单词:</label>
<input type="text" v-model="word.word" />
</div>
<div class="form-control">
<label>词性:</label>
<input type="text" v-model="word.pos" />
</div>
<div class="form-control">
<label>中文释义:</label>
<input type="text" v-model="word.definition" />
</div>
<div class="form-control">
<button type="button" @click="addWord">添加单词</button>
<button type="button" @click="clearForm">清空表单</button>
</div>
</form>
<h2>单词列表</h2>
<table>
<thead>
<tr>
<th>单词</th>
<th>词性</th>
<th>中文释义</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(word, index) in words" :key="word.id">
<td>{{ word.word }}</td>
<td>{{ word.pos }}</td>
<td>{{ word.definition }}</td>
<td>
<button type="button" @click="editWord(index)">编辑</button>
<button type="button" @click="deleteWord(index)">删除</button>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data: function() {
return {
word: {
id: null,
word: '',
pos: '',
definition: '',
},
words: [],
};
},
methods: {
addWord: function() {
if (!this.word.word || !this.word.pos || !this.word.definition) {
alert('请填写完整信息!');
return;
}
if (this.word.id === null) {
this.word.id = Date.now();
this.words.push(this.word);
} else {
const index = this.words.findIndex(w => w.id === this.word.id);
this.words.splice(index, 1, this.word);
}
this.clearForm();
},
editWord: function(index) {
const word = { ...this.words[index] };
this.word = word;
},
deleteWord: function(index) {
if (confirm(`确定删除 ${this.words[index].word} 吗?`)) {
this.words.splice(index, 1);
}
},
clearForm: function() {
this.word.id = null;
this.word.word = '';
this.word.pos = '';
this.word.definition = '';
},
},
};
</script>
<style>
.form-control {
display: flex;
margin-bottom: 10px;
}
.form-control label {
flex-basis: 100px;
}
.form-control input {
flex-grow: 1;
}
</style>
相关推荐
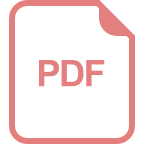
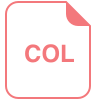
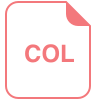













