基于python+mysql实现人事管理系统
时间: 2023-12-07 07:05:33 浏览: 77
人事管理系统是一个比较复杂的系统,需要涉及到数据库设计、用户管理、员工信息管理、考勤管理、薪资管理、绩效考核、报表查询等多个模块。下面是一个简单的人事管理系统的实现思路:
1. 数据库设计:使用MySQL数据库,设计员工信息表、考勤信息表、薪资信息表等多个表,表之间需要建立关联关系。
2. 用户管理:实现登录、注册、修改密码等功能,可以使用Python的Flask框架和MySQL连接库进行实现。
3. 员工信息管理:实现员工基本信息的录入、修改、查询和删除等功能。可以使用Python的Flask框架和MySQL连接库进行实现。
4. 考勤管理:实现考勤信息的录入、查询和统计等功能。可以使用Python的Flask框架和MySQL连接库进行实现。
5. 薪资管理:实现薪资信息的录入、查询和统计等功能。可以使用Python的Flask框架和MySQL连接库进行实现。
6. 绩效考核:实现绩效考核指标的录入、查询和统计等功能。可以使用Python的Flask框架和MySQL连接库进行实现。
7. 报表查询:实现各种报表的查询和生成。可以使用Python的Flask框架和MySQL连接库进行实现。
需要注意的是,人事管理系统的设计和实现需要考虑到数据安全、权限管理、数据一致性等问题,需要进行充分的测试和优化。
相关问题
源代码实现基于python+mysql人事管理系统
以下是一个简单的人事管理系统的Python源代码实现,使用MySQL作为数据库存储数据:
首先,需要安装MySQL的Python驱动程序,可以使用pip进行安装:
```
pip install mysql-connector-python
```
接下来,创建一个MySQL数据库,名为"hrms",并创建两个表格"employees"和"departments",分别用于存储员工和部门信息。
employees表格包含以下字段:id, name, age, gender, department_id, salary
departments表格包含以下字段:id, name, description
接着,运行以下代码实现人事管理系统:
```python
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="hrms"
)
# 定义一个函数,用于添加员工信息
def add_employee(name, age, gender, department_id, salary):
mycursor = mydb.cursor()
sql = "INSERT INTO employees (name, age, gender, department_id, salary) VALUES (%s, %s, %s, %s, %s)"
val = (name, age, gender, department_id, salary)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record inserted.")
# 定义一个函数,用于更新员工信息
def update_employee(id, name, age, gender, department_id, salary):
mycursor = mydb.cursor()
sql = "UPDATE employees SET name = %s, age = %s, gender = %s, department_id = %s, salary = %s WHERE id = %s"
val = (name, age, gender, department_id, salary, id)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) affected.")
# 定义一个函数,用于删除员工信息
def delete_employee(id):
mycursor = mydb.cursor()
sql = "DELETE FROM employees WHERE id = %s"
val = (id,)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) deleted.")
# 定义一个函数,用于查询员工信息
def get_employees():
mycursor = mydb.cursor()
sql = "SELECT employees.id, employees.name, employees.age, employees.gender, departments.name, employees.salary FROM employees INNER JOIN departments ON employees.department_id = departments.id"
mycursor.execute(sql)
result = mycursor.fetchall()
for row in result:
print(row)
# 定义一个函数,用于添加部门信息
def add_department(name, description):
mycursor = mydb.cursor()
sql = "INSERT INTO departments (name, description) VALUES (%s, %s)"
val = (name, description)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record inserted.")
# 定义一个函数,用于更新部门信息
def update_department(id, name, description):
mycursor = mydb.cursor()
sql = "UPDATE departments SET name = %s, description = %s WHERE id = %s"
val = (name, description, id)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) affected.")
# 定义一个函数,用于删除部门信息
def delete_department(id):
mycursor = mydb.cursor()
sql = "DELETE FROM departments WHERE id = %s"
val = (id,)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) deleted.")
# 定义一个函数,用于查询部门信息
def get_departments():
mycursor = mydb.cursor()
sql = "SELECT * FROM departments"
mycursor.execute(sql)
result = mycursor.fetchall()
for row in result:
print(row)
# 添加一些示例数据
add_department("IT", "Information Technology")
add_department("HR", "Human Resources")
add_employee("John Doe", 30, "Male", 1, 5000)
add_employee("Jane Smith", 25, "Female", 1, 6000)
# 查询员工信息
get_employees()
# 更新员工信息
update_employee(1, "John Smith", 31, "Male", 1, 5500)
# 删除员工信息
delete_employee(2)
# 查询部门信息
get_departments()
# 更新部门信息
update_department(1, "IT Department", "Information Technology and Services")
# 删除部门信息
delete_department(2)
# 关闭数据库连接
mydb.close()
```
以上代码实现了一个简单的人事管理系统,可以通过调用各种函数来添加、更新、删除和查询员工和部门信息。
数据库课设人事管理系统代码python
人事管理系统是一个用于管理公司员工信息的重要系统。基于Python的数据库课设人事管理系统是一个可以实现员工信息的录入、查询、修改和删除的系统。系统采用了基于MySQL数据库的数据存储,并利用了Python的各种库和框架来实现系统功能。
人事管理系统的代码主要包括以下几部分:
1. 数据库连接部分:在Python中使用MySQL的连接库和相关模块来连接数据库,进行数据的读写操作。
2. 员工信息的录入和修改部分:通过Python的界面库,用户可以输入员工的基本信息,并将信息存储到数据库中。同时,系统也提供了修改员工信息的功能,可以通过输入员工ID来对员工信息进行修改。
3. 员工信息的查询部分:利用Python的数据库操作库,可以实现各种查询功能,比如根据员工姓名、部门、职位等条件进行查询,并将结果以表格或列表的形式显示。
4. 员工信息的删除部分:可以通过输入员工ID来删除员工信息,以保证系统中的数据的准确性和完整性。
除了以上基本功能,人事管理系统的代码还可以通过Python的多线程进行并发处理,提高系统的响应速度和并发性能。另外,系统还可以实现数据的备份和恢复功能,以保证员工信息的安全和可靠性。
总之,基于Python的数据库课设人事管理系统代码可以使员工信息的管理更加方便、快捷和高效,满足公司人事管理的各种需求。
相关推荐
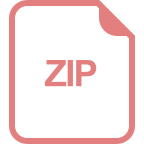
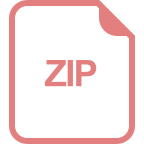
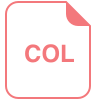
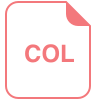
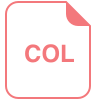
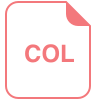
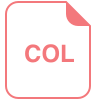







