用爬虫爬取微信公众号推文的正文内容 统计词频,计算中英文占比,给出Python代码
时间: 2024-12-15 14:13:14 浏览: 8
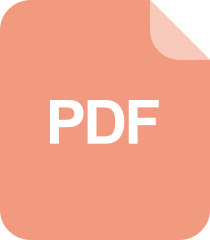
微信公众号爬虫
抓取微信公众号文章的正文内容并进行文本分析,通常需要分几个步骤:
1. **获取网页源码**:
首先,你需要使用 Python 的 requests 库来发送 HTTP 请求,通过微信的 API 或者直接访问文章页面(如:`https://mp.weixin.qq.com/s/<文章ID>`),然后解析 HTML 源码。由于微信对爬虫有反爬策略,你可能需要设置 User-Agent 和模拟浏览器行为。
```python
import requests
url = "https://mp.weixin.qq.com/s/<article_id>"
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
html_content = response.text
```
2. **提取正文**:
使用 BeautifulSoup 或 lxml 等库解析 HTML,找到包含正文的区域。这通常在 `<article>` 标签内,具体标签名可能会因设计而异。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
content_block = soup.find('div', class_='markdown-body') or soup.find('div', id='rich_media_container') # 根据实际情况修改查找标识
text_content = content_block.get_text()
```
3. **统计词频与语言占比**:
对提取出的文本进行处理,去除特殊字符、数字和标点,然后分割成单词列表。可以使用 `jieba` 库做中文分词,对于英文单词则无需特殊处理。统计词频可以用 collections.Counter。判断中英文比例可以计算中文字符数与总字符数的比例。
```python
import jieba
from collections import Counter
# 中英文分词与统计
chinese_words = [char for char in text_content if '\u4e00' <= char <= '\u9fff']
english_words = [word for word in text_content.split() if word.isalpha()]
word_counts = Counter(chinese_words + english_words)
# 计算中英文比例
chinese_ratio = len(chinese_words) / len(text_content)
```
4. **展示结果**:
可以将结果打印出来或者保存到文件中。
完整的 Python 示例代码如下:
```python
import requests
from bs4 import BeautifulSoup
import re
import jieba
from collections import Counter
# ... (上述代码)
def count_word_frequency(text):
chinese_words = [char for char in text if '\u4e00' <= char <= '\u9fff']
english_words = [word for word in text.split() if word.isalpha()]
word_counts = Counter(chinese_words + english_words)
return word_counts, chinese_ratio
word_counts, ratio = count_word_frequency(text_content)
print("词频统计:", word_counts.most_common(10)) # 输出最常见的10个词及其频率
print(f"中文占比:{ratio * 100:.2f}%")
#
阅读全文