httplib::Request
时间: 2024-10-24 13:18:09 浏览: 30
httplib::Request是Python标准库http.client模块中的一个类,它用于创建HTTP请求。这个类封装了HTTP请求的基本信息,如方法(GET、POST等)、URL、头部等,并允许设置额外的请求参数。当你使用httplib库发送HTTP请求时,通常会先实例化一个httplib::Request对象,然后调用其`request()`方法并传递给底层的socket连接。
例如:
```python
import http.client
method = 'GET'
url = 'https://example.com/api/data'
headers = {'Content-Type': 'application/json'}
req = http.client.Request(url, method=method, headers=headers)
response = http.client.HTTPConnection('example.com').getresponse()
```
在这里,`httplib::Request`充当了一个构造函数,帮助我们构建和准备发送HTTP请求。
相关问题
httplib::Client 的 Post json数据
使用 httplib 库的 Client 类可以通过 POST 请求发送 JSON 数据,具体实现如下:
```c++
#include <iostream>
#include <httplib.h>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
int main() {
// 创建 JSON 数据
json data = {
{"name", "Tom"},
{"age", 20},
{"gender", "male"}
};
// 转换为字符串
std::string json_str = data.dump();
// 创建 httplib::Client 对象
httplib::Client cli("localhost", 8080);
// 构造 POST 请求
auto res = cli.Post("/api", json_str, "application/json");
if (res) {
if (res->status == 200) {
std::cout << res->body << std::endl;
} else {
std::cout << "Error code: " << res->status << std::endl;
}
} else {
std::cout << "Error sending request." << std::endl;
}
return 0;
}
```
其中,`json` 类型是 `nlohmann/json` 库中的一种 JSON 数据类型,可以用来创建和操作 JSON 数据。在这个例子中,我们创建了一个包含名字、年龄和性别的 JSON 数据,并将其转换为字符串。
然后,我们创建了一个 `httplib::Client` 对象,并使用 `Post` 方法发送 POST 请求。第一个参数是请求的路径,第二个参数是要发送的数据,第三个参数是数据类型。
最后,我们检查响应状态码和响应体,并进行相应的处理。
类 "httplib::MultipartFormData" 没有成员 "empty"
非常抱歉,我的回答中存在错误。在cpp-httplib库中,`httplib::MultipartFormData`没有`empty`成员函数。为了获取POST请求中的文件名,你可以使用`httplib::MultipartFormData::get_file_names`函数。以下是经过修正的示例代码:
```cpp
#include <iostream>
#include "httplib.h"
int main() {
// 创建HTTP服务器
httplib::Server server;
// 处理POST请求
server.Post("/upload", [](const httplib::Request& req, httplib::Response& res) {
// 获取名为"file"的文件名
const auto& files = req.get_file_names("file");
if (!files.empty()) {
const auto& filename = files[0];
std::cout << "Received file name: " << filename << std::endl;
res.set_content("File name received", "text/plain");
return;
}
// 非法请求,返回400错误
res.status = 400;
res.set_content("Bad request", "text/plain");
});
// 启动服务器,并监听在指定端口
server.listen("localhost", 8080);
return 0;
}
```
在上面的示例中,我们使用`req.get_file_names("file")`来获取名为"file"的文件名。如果找到匹配的文件名,我们使用第一个文件名对象来获取文件名,并在控制台打印出来。
非常抱歉给你带来困扰,感谢指出错误!如果还有其他问题,请随时提问。
阅读全文
相关推荐

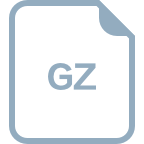













