python使用Redis缓存
时间: 2023-11-03 17:02:52 浏览: 36
1. 安装Redis
在官网上下载Redis,并安装到本地。
2. 安装redis-py
使用pip命令安装redis-py:
```
pip install redis
```
3. 连接Redis
在Python文件中,需要使用redis-py库连接到Redis:
```python
import redis
redis_client = redis.Redis(host='localhost', port=6379, db=0)
```
其中,host为Redis服务器的地址,port为端口号,db为Redis数据库的编号。
4. 设置缓存
使用set方法设置缓存:
```python
redis_client.set('key', 'value')
```
其中,key为键名,value为键值。
5. 获取缓存
使用get方法获取缓存:
```python
value = redis_client.get('key')
```
其中,key为键名,value为键值。
6. 设置缓存过期时间
使用expire方法设置缓存过期时间:
```python
redis_client.expire('key', 60)
```
其中,key为键名,60为过期时间(单位为秒)。
7. 判断缓存是否存在
使用exists方法判断缓存是否存在:
```python
if redis_client.exists('key'):
value = redis_client.get('key')
else:
value = some_function()
redis_client.set('key', value)
```
其中,key为键名,some_function()为缓存不存在时需要执行的函数。
8. 删除缓存
使用delete方法删除缓存:
```python
redis_client.delete('key')
```
其中,key为键名。
9. 清空缓存
使用flushdb方法清空缓存:
```python
redis_client.flushdb()
```
注意:该方法会删除Redis中所有的键值对,慎用。
相关推荐
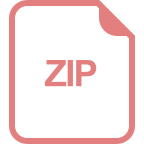
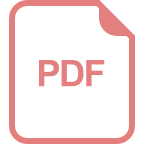














