java下载zip文件并解析成Excel 获取数据
时间: 2024-02-06 22:04:16 浏览: 24
以下是一个示例代码,演示如何使用Java下载zip文件并解析其中的Excel文件,获取数据。
```java
import java.io.*;
import java.util.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.*;
public class ZipExcelParser {
public static void main(String[] args) throws Exception {
// Step 1: 下载zip文件
String url = "https://example.com/file.zip"; // zip文件的URL
String zipFilePath = "file.zip"; // 下载后的zip文件路径
downloadFile(url, zipFilePath);
// Step 2: 解析Excel文件
String excelFilePath = null; // Excel文件路径
try (ZipInputStream zip = new ZipInputStream(new FileInputStream(zipFilePath))) {
ZipEntry entry = zip.getNextEntry();
while (entry != null) {
String fileName = entry.getName();
if (fileName.endsWith(".xlsx")) { // 只处理.xlsx文件
excelFilePath = fileName;
File file = new File(excelFilePath);
FileOutputStream fos = new FileOutputStream(file);
byte[] bytes = new byte[1024];
int length;
while ((length = zip.read(bytes)) >= 0) {
fos.write(bytes, 0, length);
}
fos.close();
break;
}
entry = zip.getNextEntry();
}
}
// Step 3: 获取Excel数据
List<List<String>> data = new ArrayList<>();
Workbook workbook = new XSSFWorkbook(new FileInputStream(excelFilePath));
Sheet sheet = workbook.getSheetAt(0); // 假设数据在第一个Sheet中
for (Row row : sheet) {
List<String> rowData = new ArrayList<>();
for (Cell cell : row) {
rowData.add(cell.toString());
}
data.add(rowData);
}
workbook.close();
// 打印数据
for (List<String> rowData : data) {
System.out.println(String.join(",", rowData));
}
}
// 下载文件
private static void downloadFile(String url, String filePath) throws Exception {
BufferedInputStream in = new BufferedInputStream(new URL(url).openStream());
FileOutputStream fos = new FileOutputStream(filePath);
byte[] dataBuffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(dataBuffer, 0, 1024)) != -1) {
fos.write(dataBuffer, 0, bytesRead);
}
fos.close();
in.close();
}
}
```
请注意,此示例代码使用了Apache POI库来解析Excel文件。如果您尚未安装它,请在项目中添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
相关推荐
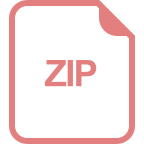
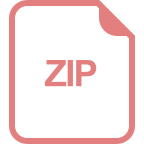












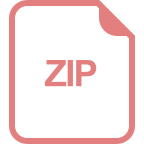
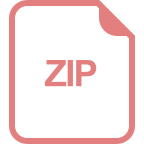