怎么才能切入sqlSession
时间: 2023-12-15 07:13:28 浏览: 44
要实现对SqlSession的切面编程,需要使用AOP框架,并定义一个切面类。下面以Spring AOP为例,介绍一下如何实现对SqlSession的切面编程。
1. 定义切面类
首先,我们需要定义一个切面类,并在其中定义一个切入点(即需要拦截的方法)。示例代码如下:
```java
@Aspect
@Component
public class SqlSessionAspect {
@Pointcut("execution(* org.apache.ibatis.session.SqlSession.*(..))")
private void sqlSessionPointcut() {}
@Before("sqlSessionPointcut()")
public void beforeSqlSession(JoinPoint joinPoint) {
// 在SqlSession执行之前执行的逻辑
// ...
}
@AfterReturning("sqlSessionPointcut()")
public void afterReturningSqlSession(JoinPoint joinPoint) {
// 在SqlSession执行成功后执行的逻辑
// ...
}
@AfterThrowing(pointcut = "sqlSessionPointcut()", throwing = "ex")
public void afterThrowingSqlSession(JoinPoint joinPoint, Throwable ex) {
// 在SqlSession执行抛出异常时执行的逻辑
// ...
}
}
```
上述代码中,我们定义了一个切面类SqlSessionAspect,并使用@Aspect和@Component注解进行标记。在切面类中,我们定义了一个切入点sqlSessionPointcut,它匹配所有SqlSession类的方法。然后,我们在该切入点上定义了三个通知(beforeSqlSession、afterReturningSqlSession和afterThrowingSqlSession),它们分别在SqlSession执行之前、执行成功后和执行抛出异常时被调用。
2. 配置AOP
接下来,我们需要在Spring配置文件中启用AOP,并将切面类SqlSessionAspect纳入切面自动代理的管理范围。示例代码如下:
```xml
<aop:aspectj-autoproxy />
<bean id="sqlSessionAspect" class="com.example.SqlSessionAspect" />
```
上述代码中,我们使用<aop:aspectj-autoproxy />标签启用了AOP,并将切面类SqlSessionAspect注册为一个Bean。
3. 使用SqlSession
现在,我们就可以在代码中使用SqlSession了。当SqlSession执行方法时,AOP框架会自动拦截它,并调用相应的通知方法。例如,下面的代码演示了如何使用SqlSession执行一条查询语句:
```java
@Autowired
private SqlSessionFactory sqlSessionFactory;
public void queryData() {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
sqlSession.selectOne("com.example.mapper.UserMapper.selectById", 1);
} finally {
sqlSession.close();
}
}
```
在上述代码中,我们使用@Autowired注解注入了SqlSessionFactory,并在queryData方法中使用SqlSession执行了一条查询语句。当SqlSession执行selectOne方法时,AOP框架会自动拦截它,并调用相应的通知方法。
相关推荐
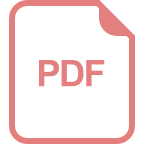
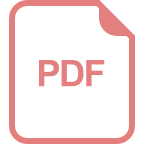
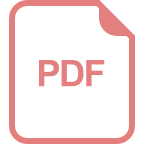














