五折交叉验证pytorch
时间: 2023-07-16 13:10:01 浏览: 297
在 PyTorch 中进行五折交叉验证的步骤如下:
1. 将数据集分成五个相等大小的子集,即数据集分成五份。
2. 创建一个循环来执行五次交叉验证。在每次循环中,选择一个子集作为验证集,其他四个子集作为训练集。
3. 对于每次循环,创建一个模型,并在训练集上训练模型。
4. 使用验证集评估模型的性能。可以计算模型的准确率、损失函数值或其他度量指标。
5. 重复步骤3和步骤4,直到每个子集都被用作验证集。
6. 将每次循环得到的性能度量进行平均,得到最终的交叉验证性能。
下面是一个简单的示例代码,演示了如何使用 PyTorch 进行五折交叉验证:
```python
import torch
import torch.nn as nn
from sklearn.model_selection import KFold
# 定义模型
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
# 定义模型结构
def forward(self, x):
# 前向传播逻辑
return x
# 创建数据集和标签
dataset = ...
labels = ...
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001)
# 创建五折交叉验证对象
kfold = KFold(n_splits=5, shuffle=True)
# 开始交叉验证
for train_indices, val_indices in kfold.split(dataset):
# 将数据集分成训练集和验证集
train_dataset = dataset[train_indices]
train_labels = labels[train_indices]
val_dataset = dataset[val_indices]
val_labels = labels[val_indices]
# 创建模型实例
model = MyModel()
# 训练模型
for epoch in range(num_epochs):
# ...
# 前向传播、计算损失、反向传播、更新参数等
# 在验证集上评估模型
# ...
# 计算性能度量指标
# 打印当前交叉验证的性能指标
print('Validation performance:', performance_metric)
```
需要注意的是,以上代码只是一个示例,具体实现可能需要根据你的数据集和模型进行适当的修改。此外,你可能需要根据具体情况选择合适的性能度量指标和优化器等。
阅读全文
相关推荐
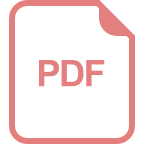
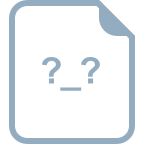

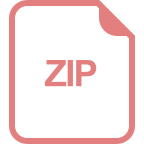
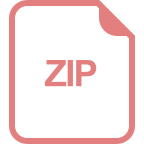
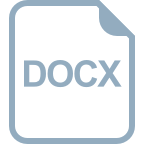