asp.net Authentication
时间: 2023-12-12 07:35:04 浏览: 108
ASP.NET身份验证是一种用于验证用户身份的机制,它可以确保只有经过身份验证的用户才能访问应用程序的受保护部分。ASP.NET Core提供了多种身份验证方式,包括Cookie身份验证、JWT身份验证、OpenID Connect身份验证等。其中,Cookie身份验证是最常用的一种方式。
以下是ASP.NET Core中使用Cookie身份验证的示例代码:
1. 在Startup.cs文件的ConfigureServices方法中添加身份验证服务:
```csharp
services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme)
.AddCookie(options =>
{
options.LoginPath = "/Account/Login";
options.LogoutPath = "/Account/Logout";
});
```
2. 在Startup.cs文件的Configure方法中启用身份验证中间件:
```csharp
app.UseAuthentication();
```
3. 在需要进行身份验证的Controller或Action上添加[Authorize]特性:
```csharp
[Authorize]
public class HomeController : Controller
{
// ...
}
```
4. 在登录Controller中使用SignInAsync方法进行登录:
```csharp
public async Task<IActionResult> Login(LoginViewModel model)
{
// 验证用户名和密码
if (/* 验证通过 */)
{
// 创建用户标识
var claims = new List<Claim>
{
new Claim(ClaimTypes.Name, model.UserName)
};
var identity = new ClaimsIdentity(claims, CookieAuthenticationDefaults.AuthenticationScheme);
// 登录
await HttpContext.SignInAsync(CookieAuthenticationDefaults.AuthenticationScheme, new ClaimsPrincipal(identity));
// 跳转到首页
return RedirectToAction("Index", "Home");
}
// 验证失败,返回登录页面
return View(model);
}
```
5. 在注销Controller中使用SignOutAsync方法进行注销:
```csharp
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync(CookieAuthenticationDefaults.AuthenticationScheme);
return RedirectToAction("Index", "Home");
}
```
阅读全文
相关推荐
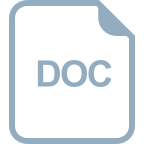
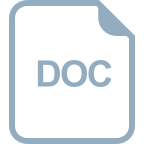
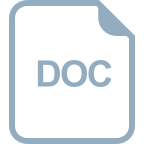
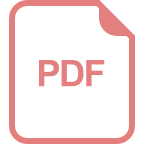
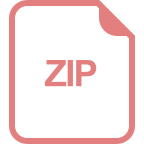
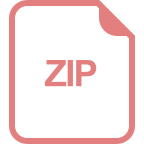
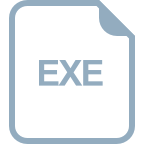
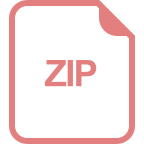
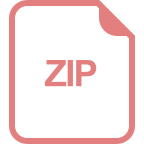
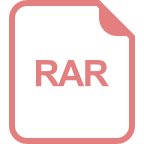
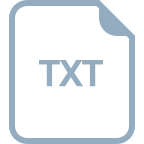
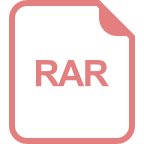
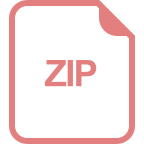
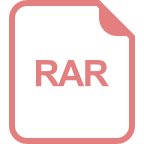
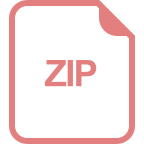
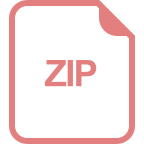
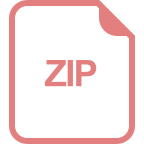
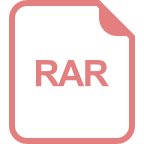
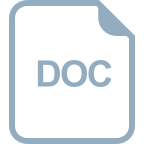