编程题:线程任务传参实现: 传字符串描述线程的任务+时间片C语言
时间: 2024-10-19 10:11:16 浏览: 24
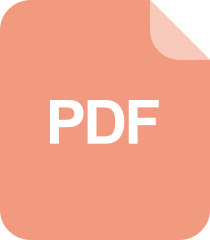
招银网络校园招聘历年经典面试题汇总:C++研发岗1
在C语言中,由于其线程模型相对基础,并没有内置的`Thread`或类似功能可以直接提供带参数的任务描述和时间片设置。然而,你可以通过创建自定义的数据结构来封装任务信息,并利用互斥量(mutexes)或信号量(semaphores)来传递和同步数据。
下面是一个简单的示例,展示如何使用C标准库中的`pthread`函数来创建一个线程并传入字符串参数作为任务描述:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 定义任务结构体,包含任务描述和时间片
typedef struct {
char* task_description;
int time_slice; // 假设时间片单位为毫秒
} Task;
void *thread_function(void *arg) {
// 解析传入的任务参数
Task* task = (Task*) arg;
printf("Starting thread with task '%s' and time slice %d ms.\n", task->task_description, task->time_slice);
// 这里只是一个演示,实际任务应该替换为你的业务逻辑
// 模拟任务处理过程
sleep(task->time_slice / 1000); // 睡眠指定的时间
pthread_exit(NULL); // 结束线程
}
int main() {
Task task = {"我的任务", 5000}; // 示例任务,5秒时间片
// 创建新线程
pthread_t thread_id;
if (pthread_create(&thread_id, NULL, thread_function, &task)) {
perror("Error creating thread");
exit(1);
}
// 等待线程结束
pthread_join(thread_id, NULL);
free(task.task_description); // 释放任务描述内存
return 0;
}
```
在这个例子中,`main`函数创建了一个新的线程,并将`task`结构体指针传递给`thread_function`。每个线程会根据接收到的`task_description`和`time_slice`执行相应的操作。
请注意,这个示例非常简化,实际应用中可能会有更复杂的任务管理和线程控制机制。
阅读全文
相关推荐
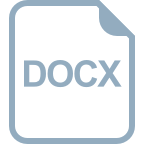
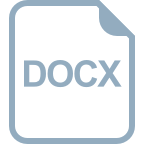
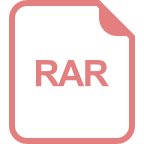
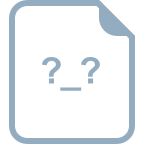
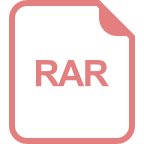
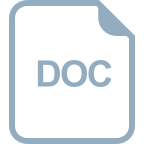
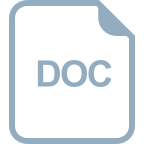
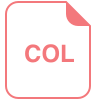
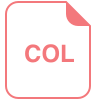
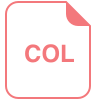
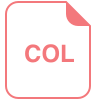
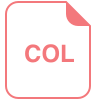
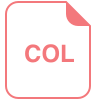
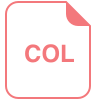
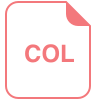
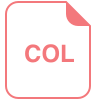
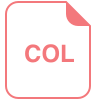
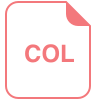