springboot sftp文件读取
时间: 2023-11-15 15:02:06 浏览: 54
在Spring Boot项目中,可以使用JSch库来实现SFTP文件读取。具体步骤如下:
1. 在pom.xml文件中添加JSch依赖。
2. 在application.yaml配置文件中配置SFTP连接信息,包括IP地址、端口号、用户名、密码和文件根目录等。
3. 创建SftpUtil类,使用JSch库连接SFTP服务器,并实现文件上传、下载、删除等操作。
4. 在需要使用SFTP文件的地方,调用SftpUtil类中的方法即可。
需要注意的是,使用SFTP协议需要保证服务器端已经安装了SFTP服务,并且开启了SFTP端口。同时,需要保证本地环境中已经安装了JSch库。
相关问题
springboot sftp文件批量上传
以下是使用Spring Boot实现SFTP文件批量上传的示例代码:
```java
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.StringUtils;
import java.io.File;
import java.util.Vector;
@Component
public class SftpUtil {
@Autowired
private SftpConfig sftpConfig;
/**
* 批量上传文件
*
* @param directory 上传的目录
* @param uploadFiles 待上传的文件列表
* @return
*/
public boolean batchUpload(String directory, Vector<File> uploadFiles) {
ChannelSftp sftp = null;
Session session = null;
try {
JSch jsch = new JSch();
session = jsch.getSession(sftpConfig.getUsername(), sftpConfig.getHost(), sftpConfig.getPort());
session.setPassword(sftpConfig.getPassword());
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
sftp = (ChannelSftp) session.openChannel("sftp");
sftp.connect();
if (!StringUtils.isEmpty(directory)) {
sftp.cd(directory);
}
for (File file : uploadFiles) {
sftp.put(file.getAbsolutePath(), file.getName());
}
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
} finally {
if (sftp != null) {
sftp.disconnect();
}
if (session != null) {
session.disconnect();
}
}
}
}
```
在上述代码中,我们使用了JSch库来连接SFTP服务器,并使用ChannelSftp对象进行文件上传。具体实现中,我们通过传入上传目录和待上传文件列表来实现批量上传。在上传之前,我们需要先连接SFTP服务器,并切换到指定的上传目录。
springboot sftp csv读取
在Spring Boot中使用SFTP协议来读取CSV文件可以通过以下步骤实现。
首先,我们需要添加所需的依赖项。在pom.xml文件中添加以下依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-integration</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-sftp</artifactId>
</dependency>
<dependency>
<groupId>net.schmizz</groupId>
<artifactId>sshj</artifactId>
<version>0.27.0</version>
</dependency>
```
接下来,我们需要设置SFTP连接的配置信息。可以在application.properties文件中添加以下属性:
```
spring.integration.sftp.host=your-sftp-host
spring.integration.sftp.username=your-sftp-username
spring.integration.sftp.password=your-sftp-password
```
然后,我们可以编写一个服务类来读取SFTP上的CSV文件。可以使用`SftpInboundFileSynchronizer`来实现SFTP文件同步,使用`SftpInboundFileSynchronizingMessageSource`来生成SFTP文件输入端点。
在服务类中,我们可以定义一个方法来读取SFTP上的CSV文件:
```java
@Service
public class SftpService {
@Value("${spring.integration.sftp.remote.directory}")
private String sftpRemoteDirectory;
@Bean
public SftpInboundFileSynchronizer sftpInboundFileSynchronizer() {
SftpInboundFileSynchronizer synchronizer = new SftpInboundFileSynchronizer(sessionFactory());
synchronizer.setDeleteRemoteFiles(false);
synchronizer.setRemoteDirectory(sftpRemoteDirectory);
return synchronizer;
}
@Bean
@InboundChannelAdapter(channel = "sftpChannel", poller = @Poller(fixedDelay = "5000"))
public MessageSource<File> sftpMessageSource() {
SftpInboundFileSynchronizingMessageSource source = new SftpInboundFileSynchronizingMessageSource(sftpInboundFileSynchronizer());
source.setLocalDirectory(new File("local-directory"));
source.setAutoCreateLocalDirectory(true);
source.setLocalFilter(new AcceptOnceFileListFilter<File>());
return source;
}
@ServiceActivator(inputChannel = "sftpChannel")
public void handleFile(Message<File> message) {
File file = message.getPayload();
// 读取CSV文件的逻辑
}
}
```
在上述代码中,`sftpRemoteDirectory`属性为SFTP上的远程目录,`sftpInboundFileSynchronizer`方法用于创建SFTP文件同步器,`sftpMessageSource`方法用于创建SFTP文件输入端点。 `handleFile`方法用于处理读取到的CSV文件。
最后,我们可以在Spring Boot应用中使用这个服务类来读取SFTP上的CSV文件。可以通过调用`handleFile`方法来触发读取文件的操作。
以上就是使用Spring Boot来读取SFTP上的CSV文件的简单实现。希望能对你有所帮助。
相关推荐
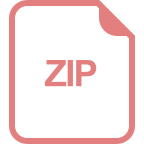
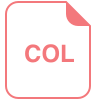
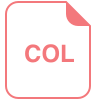
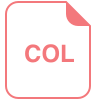
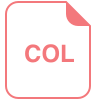
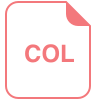







