请编制C程序,利用线性表的链式存储方式实现下列功能,建立线性表、输出线性表、在线性表的第i个元素之前插入一个元素、删除线性表中第i个元素、在线性表中查找值为x的元素并返回查找结果、将两个有序链表合并为一个有序链表。
时间: 2024-05-01 13:16:13 浏览: 9
非常感谢您的提问。以下是对您的问题的回答:
以下是C语言程序,利用线性表的链式存储方式实现建立线性表、输出线性表、在线性表的第i个元素之前插入一个元素、删除线性表中第i个元素、在线性表中查找值为x的元素并返回查找结果、将两个有序链表合并为一个有序链表的功能:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* create(int n) {
struct Node *head, *p, *node;
head = (struct Node*)malloc(sizeof(struct Node));
p = head;
for (int i = 0; i < n; ++i) {
node = (struct Node*)malloc(sizeof(struct Node));
printf("请输入第 %d 个元素的值:", i + 1);
scanf("%d", &node->data);
p->next = node;
p = node;
}
p->next = NULL;
return head;
}
void display(struct Node* head) {
struct Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void insert(struct Node* head, int i, int x) {
struct Node *p, *node;
p = head;
for (int j = 0; j < i - 1; ++j) {
p = p->next;
}
node = (struct Node*)malloc(sizeof(struct Node));
node->data = x;
node->next = p->next;
p->next = node;
}
void removeNode(struct Node* head, int i) {
struct Node *p, *q;
p = head;
for (int j = 0; j < i - 1; ++j) {
p = p->next;
}
q = p->next;
p->next = q->next;
free(q);
}
struct Node* search(struct Node* head, int x) {
struct Node* p = head->next;
while (p != NULL) {
if (p->data == x) {
return p;
}
p = p->next;
}
return NULL;
}
struct Node* merge(struct Node* list1, struct Node* list2) {
struct Node *p = list1->next, *q = list2->next, *result, *s;
result = (struct Node*)malloc(sizeof(struct Node));
s = result;
while (p != NULL && q != NULL) {
if (p->data <= q->data) {
s->next = p;
p = p->next;
}
else {
s->next = q;
q = q->next;
}
s = s->next;
}
if (p != NULL) {
s->next = p;
}
if (q != NULL) {
s->next = q;
}
return result;
}
int main() {
int n, i, x;
struct Node *list1, *list2, *result;
printf("请输入线性表的长度:");
scanf("%d", &n);
list1 = create(n);
printf("线性表的元素为:");
display(list1);
printf("请输入要插入的元素的位置和值:");
scanf("%d %d", &i, &x);
insert(list1, i, x);
printf("插入后的线性表为:");
display(list1);
printf("请输入要删除元素的位置:");
scanf("%d", &i);
removeNode(list1, i);
printf("删除后的线性表为:");
display(list1);
printf("请输入要查找的元素的值:");
scanf("%d", &x);
struct Node* node = search(list1, x);
if (node == NULL) {
printf("未找到 %d\n", x);
}
else {
printf("%d 的位置为:%ld\n", x, node - list1);
}
printf("请输入有序链表 1 的长度:");
scanf("%d", &n);
list1 = create(n);
printf("请输入有序链表 2 的长度:");
scanf("%d", &n);
list2 = create(n);
result = merge(list1, list2);
printf("合并后的有序链表为:");
display(result);
return 0;
}
```
如果您有任何其他问题,请随时向我提问。感谢您的支持!
相关推荐
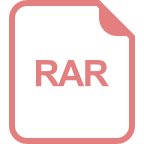














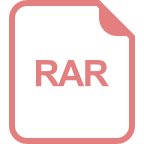