请给出决策树算法详细内容及步骤,且使用python代码实现,并给出详细注释和步骤解释
时间: 2024-04-16 09:24:28 浏览: 22
决策树算法是一种基于树结构的监督学习算法,它可以用于分类和回归任务。下面是决策树算法的详细步骤和使用Python代码实现的示例。
步骤1: 导入所需的库
```python
import numpy as np
import pandas as pd
```
步骤2: 定义决策树节点类
```python
class Node:
def __init__(self, feature_idx=None, threshold=None, value=None, left=None, right=None):
self.feature_idx = feature_idx # 用于分割数据集的特征索引
self.threshold = threshold # 分割阈值
self.value = value # 叶节点的预测值
self.left = left # 左子节点
self.right = right # 右子节点
```
步骤3: 定义决策树算法函数
```python
def decision_tree(X, y):
# 创建根节点
root = build_tree(X, y)
return root
def build_tree(X, y):
# 创建节点并选择最佳分割特征和阈值
feature_idx, threshold = choose_split(X, y)
# 如果无法再分割,则返回叶节点
if feature_idx is None or threshold is None:
return Node(value=np.mean(y))
# 根据最佳分割特征和阈值划分数据集
X_left, y_left, X_right, y_right = split_data(X, y, feature_idx, threshold)
# 递归构建左子树和右子树
left = build_tree(X_left, y_left)
right = build_tree(X_right, y_right)
# 创建当前节点
return Node(feature_idx=feature_idx, threshold=threshold, left=left, right=right)
```
步骤4: 定义选择最佳分割特征和阈值的函数
```python
def choose_split(X, y):
best_feature_idx = None
best_threshold = None
best_gini = float('inf')
# 遍历每个特征
for feature_idx in range(X.shape[1]):
unique_values = np.unique(X[:, feature_idx])
# 尝试每个特征值作为阈值
for threshold in unique_values:
# 根据特征值分割数据集
X_left, y_left, X_right, y_right = split_data(X, y, feature_idx, threshold)
# 计算基尼指数
gini = gini_index(y_left, y_right)
# 更新最佳分割特征和阈值
if gini < best_gini:
best_gini = gini
best_feature_idx = feature_idx
best_threshold = threshold
return best_feature_idx, best_threshold
```
步骤5: 定义数据集分割函数
```python
def split_data(X, y, feature_idx, threshold):
mask = X[:, feature_idx] <= threshold
X_left, y_left = X[mask], y[mask]
X_right, y_right = X[~mask], y[~mask]
return X_left, y_left, X_right, y_right
```
步骤6: 定义基尼指数计算函数
```python
def gini_index(y_left, y_right):
n_left = len(y_left)
n_right = len(y_right)
n_total = n_left + n_right
gini_left = 1 - np.sum(np.square(np.bincount(y_left) / n_left))
gini_right = 1 - np.sum(np.square(np.bincount(y_right) / n_right))
gini = (n_left / n_total) * gini_left + (n_right / n_total) * gini_right
return gini
```
步骤7: 使用示例数据集进行训练和预测
```python
X = np.array([[1, 1], [1, 0], [0, 1], [0, 0]])
y = np.array([1, 1, 0, 0])
# 构建决策树
tree = decision_tree(X, y)
# 预测新样本
new_sample = np.array([[1, 0]])
prediction = predict(tree, new_sample)
print("预测结果:", prediction)
```
以上是决策树算法的详细步骤和使用Python代码实现的示例。注意,这只是一个简单的示例,实际应用中可能需要考虑更多的优化和细节处理。
相关推荐
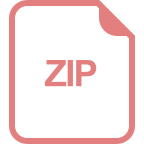
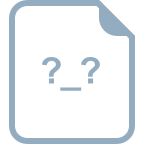
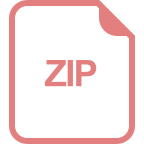














