setrequestProperty函数用法
时间: 2023-11-19 11:06:45 浏览: 28
`setRequestProperty` 函数是 `URLConnection` 类的一个方法,用于设置 HTTP 请求头属性。它的语法如下:
```
public void setRequestProperty(String key, String value)
```
其中,`key` 是要设置的 HTTP 请求头属性的键,`value` 是对应的值。可以多次调用 `setRequestProperty` 方法来设置多个请求头属性。
例如,下面的代码设置了一个 `User-Agent` 请求头属性:
```java
URL url = new URL("https://www.example.com");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("User-Agent", "Mozilla/5.0");
```
这样,当发送 HTTP 请求时,会在请求头中包含一个 `User-Agent` 属性,其值为 `Mozilla/5.0`。
相关问题
将post请求封装成Java函数
### 回答1:
可以尝试使用Apache HttpClient来封装post请求为Java函数,具体的步骤可以参考官方文档中的例子:http://hc.apache.org/httpclient-3.x/tutorial.html#Using%20the%20POST%20method
### 回答2:
将 post 请求封装为 Java 函数可以通过使用 Java 的 HttpURLConnection 类来实现。以下是一个基本的封装函数的示例:
```java
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostRequest {
public static String sendPostRequest(String url, String data) throws IOException {
HttpURLConnection connection = null;
BufferedReader reader = null;
try {
// 创建 URL 对象
URL postUrl = new URL(url);
// 打开连接
connection = (HttpURLConnection) postUrl.openConnection();
// 设置请求方法为 POST
connection.setRequestMethod("POST");
connection.setDoOutput(true);
// 设置请求体的内容类型为 application/json
connection.setRequestProperty("Content-Type", "application/json");
// 发送 POST 请求的正文
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
outputStream.writeBytes(data);
outputStream.flush();
outputStream.close();
// 获取响应的状态码
int responseCode = connection.getResponseCode();
// 读取响应结果
reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
// 返回响应结果
return response.toString();
} finally {
if (reader != null) {
reader.close();
}
if (connection != null) {
connection.disconnect();
}
}
}
}
```
在上面的示例中,我们首先创建了一个 URL 对象,然后打开连接并设置请求方法为 POST。我们还设置了请求体的内容类型为 application/json。接下来,我们将请求数据写入请求正文,并发送请求。然后我们获取响应的状态码,然后使用 BufferedReader 读取响应结果并将其存储到 StringBuilder 对象中。最后,我们返回响应结果。
你可以通过调用 `sendPostRequest(url, data)` 的方式来使用该函数,其中 `url` 代表请求的 URL,`data` 代表请求的数据。
需要注意的是,上述示例中的代码只是一个简单的封装示例,并且假设请求的数据是以字符串形式传递的。实际上,我们可能会在数据的基础上进行一系列的操作,例如序列化、加密等。在实际使用中,我们需要根据具体的需求进行适当的调整和优化。
### 回答3:
将post请求封装成Java函数的过程需要使用Java中的HttpURLConnection类和BufferedReader类来实现。Java函数可以包括以下步骤:
1. 创建URL对象,指定要发送post请求的地址。
2. 打开URL连接,得到HttpURLConnection对象。
3. 设置请求参数和请求头,如设置请求方法为post、设置Content-Type为application/json等。
4. 获取输出流,通过输出流向服务器发送post请求的参数和数据。
5. 获取响应码,通过HttpURLConnection对象的getResponseCode()方法。
6. 根据响应码判断请求是否成功,如果成功则获取输入流。
7. 创建BufferedReader对象,读取服务器返回的数据。
8. 将服务器返回的数据保存到StringBuilder中,通过循环读取并拼接字符串。
9. 关闭输入流和输出流,释放资源。
10. 返回获取到的服务器返回数据。
以下是一个简单的示例代码:
```
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostRequestExample {
public static String sendPostRequest(String url, String params) {
StringBuilder response = new StringBuilder();
try {
URL postUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) postUrl.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setDoOutput(true);
OutputStream outputStream = connection.getOutputStream();
outputStream.write(params.getBytes("UTF-8"));
outputStream.flush();
outputStream.close();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
}
connection.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
return response.toString();
}
public static void main(String[] args) {
String url = "http://example.com/api";
String params = "key1=value1&key2=value2";
String result = sendPostRequest(url, params);
System.out.println(result);
}
}
```
以上示例代码封装了一个名为sendPostRequest的函数,接受一个url和params参数,通过post请求发送数据,并返回服务器返回的数据。在main函数中调用sendPostRequest函数并传入示例的url和params即可执行post请求。
用Java写一个上传文件的功能函数
好的,以下是使用 Java 上传文件的示例代码:
```
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Base64;
import java.util.Map;
public class FileUploader {
private static final String LINE_FEED = "\r\n";
/**
* 使用 POST 方法上传文件
*
* @param requestURL 请求的 URL
* @param fileFormName 文件表单字段的名称
* @param file 文件对象
* @param authHeader 认证的头信息(可选)
* @param otherParams 其他参数(可选)
* @return 服务器的响应信息
* @throws Exception
*/
public static String uploadFile(String requestURL, String fileFormName, File file,
String authHeader, Map<String, String> otherParams) throws Exception {
HttpURLConnection connection = null;
OutputStream outputStream = null;
BufferedReader reader = null;
try {
// 创建连接
URL url = new URL(requestURL);
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoInput(true);
connection.setDoOutput(true);
connection.setUseCaches(false);
connection.setRequestProperty("Connection", "Keep-Alive");
connection.setRequestProperty("Content-Type", "multipart/form-data;boundary=" + getBoundary());
// 设置认证的头信息
if (authHeader != null && !authHeader.isEmpty()) {
connection.setRequestProperty("Authorization", authHeader);
}
// 获取输出流
outputStream = connection.getOutputStream();
// 写入文件数据
outputStream.write(("--" + getBoundary() + LINE_FEED).getBytes());
outputStream.write(("Content-Disposition: form-data; name=\"" + fileFormName + "\"; filename=\""
+ file.getName() + "\"" + LINE_FEED).getBytes());
outputStream.write(("Content-Type: " + URLConnection.guessContentTypeFromName(file.getName()) + LINE_FEED)
.getBytes());
outputStream.write(("Content-Transfer-Encoding: binary" + LINE_FEED).getBytes());
outputStream.write(LINE_FEED.getBytes());
FileInputStream inputStream =
相关推荐
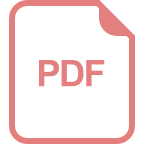
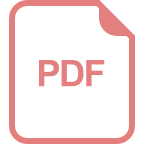
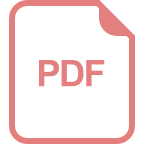








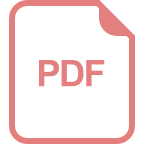
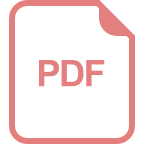
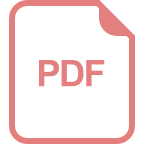
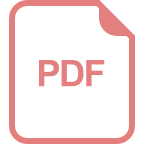
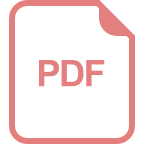