veins中实现信道选择和优化延迟的代码
时间: 2024-02-17 12:02:31 浏览: 35
在Veins中,信道选择和优化延迟的实现涉及到许多不同的组件和模块。以下是一些可能有用的参考资料和代码片段:
1. 在Veins中,车辆可以使用Omnet++的信道模型选择不同的信道进行通信。您可以使用车辆当前位置和传输距离等信息来选择最佳信道。以下是一个简单的示例:
```
void MyVeinsApp::sendMessage()
{
// Get the current position of the vehicle
Coord currentPosition = mobility->getCurrentPosition();
// Find the closest access point
AccessPoint closestAP;
double minDistance = std::numeric_limits<double>::max();
for (const auto& ap : accessPoints) {
double distance = currentPosition.distance(ap.getPosition());
if (distance < minDistance) {
minDistance = distance;
closestAP = ap;
}
}
// Select the channel with the least interference
double minInterference = std::numeric_limits<double>::max();
int bestChannel = -1;
for (int i = 0; i < numChannels; i++) {
double interference = calculateInterference(i, closestAP);
if (interference < minInterference) {
minInterference = interference;
bestChannel = i;
}
}
// Transmit the message on the selected channel
sendOnChannel(bestChannel);
}
```
2. 为了优化延迟,您可以使用Veins中的消息调度程序来控制消息发送和接收的时间。您可以使用以下代码来指定消息的发送时间和接收时间:
```
void MyVeinsApp::sendMessage()
{
// ...
// Schedule the message transmission
simtime_t sendTime = simTime() + delay;
sendMessageAt(sendTime);
// Schedule the message reception
simtime_t receiveTime = sendTime + transmissionTime;
scheduleAt(receiveTime, &MyVeinsApp::receiveMessage);
}
void MyVeinsApp::receiveMessage()
{
// ...
// Process the message
}
```
3. 如果您需要更复杂的信道选择和优化延迟算法,可以考虑使用Veins中的Mobility和TraCIScenarioManager模块。这些模块允许您访问车辆和道路拓扑结构,并使用更高级的算法来选择信道和优化延迟。以下是一个示例:
```
void MyVeinsApp::sendMessage()
{
// Get the current position of the vehicle
Coord currentPosition = mobility->getCurrentPosition();
// Find the closest access point
AccessPoint closestAP = findClosestAccessPoint(currentPosition);
// Select the channel with the least interference
int bestChannel = selectBestChannel(closestAP);
// Schedule the message transmission
simtime_t sendTime = calculateSendTime(currentPosition, closestAP, bestChannel);
sendMessageAt(sendTime);
// Schedule the message reception
simtime_t receiveTime = calculateReceiveTime(sendTime, currentPosition, closestAP);
scheduleAt(receiveTime, &MyVeinsApp::receiveMessage);
}
AccessPoint MyVeinsApp::findClosestAccessPoint(Coord position)
{
// Find the closest access point using the TraCIScenarioManager
std::vector<AccessPoint> accessPoints = traci->getAccessPoints();
AccessPoint closestAP;
double minDistance = std::numeric_limits<double>::max();
for (const auto& ap : accessPoints) {
double distance = position.distance(ap.getPosition());
if (distance < minDistance) {
minDistance = distance;
closestAP = ap;
}
}
return closestAP;
}
int MyVeinsApp::selectBestChannel(AccessPoint ap)
{
// Select the channel with the least interference
int bestChannel = -1;
double minInterference = std::numeric_limits<double>::max();
for (int i = 0; i < numChannels; i++) {
double interference = calculateInterference(i, ap);
if (interference < minInterference) {
minInterference = interference;
bestChannel = i;
}
}
return bestChannel;
}
simtime_t MyVeinsApp::calculateSendTime(Coord currentPosition, AccessPoint ap, int channel)
{
// Calculate the transmission delay based on the distance to the access point
double distance = currentPosition.distance(ap.getPosition());
simtime_t delay = calculateDelay(distance);
// Add a random offset to the delay to prevent collisions
delay += uniform(0, maxDelayOffset);
// Calculate the time when the message should be sent
simtime_t sendTime = simTime() + delay;
// Set the channel for the message
setChannel(channel);
return sendTime;
}
simtime_t MyVeinsApp::calculateReceiveTime(simtime_t sendTime, Coord currentPosition, AccessPoint ap)
{
// Calculate the transmission time based on the distance to the access point
double distance = currentPosition.distance(ap.getPosition());
simtime_t transmissionTime = calculateTransmissionTime(distance);
// Calculate the time when the message should be received
simtime_t receiveTime = sendTime + transmissionTime;
return receiveTime;
}
```
这只是一个简单的示例,您可能需要根据您的具体需求进行调整。希望这能对您有所帮助!
阅读全文
相关推荐
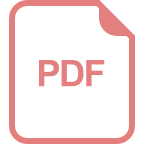
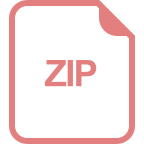
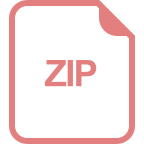



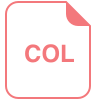
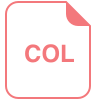
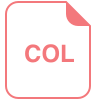
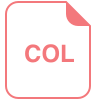
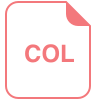






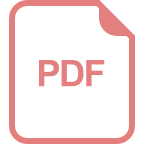