Transformer 翻译成汉语
时间: 2024-12-29 18:25:11 浏览: 6
### Transformer 术语及其对应的中文翻译
#### 输入嵌入层 (Input Embedding)
输入嵌入层负责将每个单词转换成一个稠密的向量表示,这些向量捕捉了词语的语义信息[^2]。
```python
import torch.nn as nn
class InputEmbedding(nn.Module):
def __init__(self, vocab_size, d_model):
super(InputEmbedding, self).__init__()
self.embedding = nn.Embedding(vocab_size, d_model)
def forward(self, x):
return self.embedding(x) * math.sqrt(d_model)
```
#### 位置编码 (Positional Encoding)
为了使模型能够区分同一词汇在不同位置的意义差异,在输入嵌入的基础上加入了位置编码。位置编码使用正余弦函数来标记每个词的位置信息。
```python
def positional_encoding(position, d_model):
angle_rads = get_angles(np.arange(position)[:, np.newaxis],
np.arange(d_model)[np.newaxis, :],
d_model)
sines = np.sin(angle_rads[:, 0::2])
cosines = np.cos(angle_rads[:, 1::2])
pos_encoding = np.concatenate([sines, cosines], axis=-1)
pos_encoding = pos_encoding[np.newaxis, ...]
return tf.cast(pos_encoding, dtype=tf.float32)
```
#### 自注意力机制 (Self-Attention Mechanism)
自注意力机制用于衡量上下文中每一个单词对于当前处理单词的重要性程度。这种机制允许网络关注整个句子中的所有其他部分而不仅仅是相邻的部分[^1]。
```python
def scaled_dot_product_attention(q, k, v, mask=None):
matmul_qk = tf.matmul(q, k, transpose_b=True)
dk = tf.cast(tf.shape(k)[-1], tf.float32)
logits = matmul_qk / tf.math.sqrt(dk)
if mask is not None:
logits += (mask * -1e9)
attention_weights = tf.nn.softmax(logits, axis=-1)
output = tf.matmul(attention_weights, v)
return output, attention_weights
```
#### 编码器 (Encoder)
编码器由多个相同的层堆叠而成,每一层包含了两个子层:一个多头自注意分子层和一个全连接前馈神经网络。这两个子层都采用了残差连接并进行了标准化操作。
#### 解码器 (Decoder)
解码器同样是由多层相同结构组成,不过除了包含与编码器类似的两种子层外还额外增加了一种特殊的掩蔽多头自注意力机制,防止当前位置看到未来的信息。
阅读全文
相关推荐


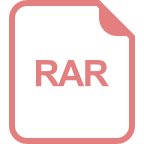

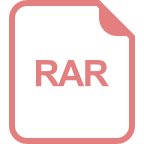












