def get_img_url(response):
时间: 2023-09-12 22:07:49 浏览: 45
这是一个Python函数的定义,函数名为`get_img_url`,它接受一个参数`response`。该函数的作用是从HTTP响应中解析出图片的URL。
假设你已经发送了一个HTTP请求,并使用response变量保存了响应,你可以将response传递给get_img_url函数来解析图像URL。以下是一个示例函数实现:
```
def get_img_url(response):
# 判断响应是否成功
if response.status_code == 200:
# 解析响应,获取图片的URL
img_url = response.json()['img_url']
# 返回图片的URL
return img_url
else:
# 如果响应不成功,返回None
return None
```
这个函数假定响应是一个JSON格式的文本响应,其中包含一个名为`img_url`的键,该键的值是图像的URL。如果响应成功,该函数将返回此URL,否则将返回`None`。
相关问题
class PicSpiderPipeline(ImagesPipeline): def get_media_requests(self, item, info): image_requests = super().get_media_requests(item,info) for img_req in image_requests: img_req.item = item return image_requests def file_path(self, request, response=None, info=None, *, item=None): old_path = super().file_path(request,response,info) title = request.item['title'] save_path = os.path.join(settings.IMAGES_STORE,title[0].replace('|','')) image_name = old_path.replace('full/','') return os.path.join(save_path,image_name) 此代码中怎样知道标题title含有特殊符号
在给`item`赋值的时候,可以使用`re.sub()`函数来替换特殊字符。以下是修改后的代码:
```python
import re
class PicSpiderPipeline(ImagesPipeline):
def get_media_requests(self, item, info):
image_requests = super().get_media_requests(item, info)
for img_req in image_requests:
img_req.item = item
return image_requests
def file_path(self, request, response=None, info=None, *, item=None):
old_path = super().file_path(request, response, info)
title = request.item['title']
# 使用re.sub()替换特殊字符
title = re.sub(r'[|]', '', title)
save_path = os.path.join(settings.IMAGES_STORE, title[0])
image_name = old_path.replace('full/', '')
return os.path.join(save_path, image_name)
```
在这个修改后的代码中,通过使用`re.sub()`函数,我们将标题中的`|`符号替换为空字符串,这样就可以确保标题中不再含有特殊字符。然后我们仍然使用替换后的标题作为保存路径的一部分。
img_download(self, img_url):
这个函数的作用是下载图片。它接受一个参数img_url,表示图片的链接地址。
函数的具体实现可能包括以下步骤:
1. 使用Python的requests库发送HTTP请求,获取图片的二进制数据;
2. 将二进制数据保存到本地文件中,可以使用Python的open()函数和write()方法实现。
下面是一个简单的实现示例:
```python
import requests
def img_download(img_url):
response = requests.get(img_url)
if response.status_code == 200:
with open('image.jpg', 'wb') as f:
f.write(response.content)
print('图片下载成功')
else:
print('图片下载失败')
```
这个函数会将img_url所指向的图片下载到当前工作目录下的image.jpg文件中。如果下载成功,会输出"图片下载成功",否则输出"图片下载失败"。
相关推荐
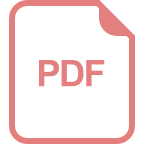
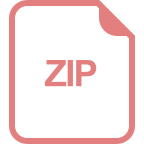









