java字符串数组进行二叉树堆排序
时间: 2023-06-19 10:08:55 浏览: 121
首先,需要定义一个二叉堆类,包含堆的初始化、插入、删除等方法。然后,将字符串数组插入到堆中,最后依次从堆中删除元素,即可得到排序后的字符串数组。
以下是一个示例代码:
```java
public class BinaryHeap {
private String[] heapArray;
private int maxSize;
private int currentSize;
public BinaryHeap(int maxSize) {
this.maxSize = maxSize;
this.currentSize = 0;
this.heapArray = new String[maxSize];
}
public boolean isEmpty() {
return currentSize == 0;
}
public boolean insert(String value) {
if (currentSize == maxSize) {
return false;
}
heapArray[currentSize] = value;
trickleUp(currentSize++);
return true;
}
public String remove() {
String root = heapArray[0];
heapArray[0] = heapArray[--currentSize];
trickleDown(0);
return root;
}
private void trickleUp(int index) {
int parent = (index - 1) / 2;
String bottom = heapArray[index];
while (index > 0 && heapArray[parent].compareTo(bottom) < 0) {
heapArray[index] = heapArray[parent];
index = parent;
parent = (parent - 1) / 2;
}
heapArray[index] = bottom;
}
private void trickleDown(int index) {
int largerChild;
String top = heapArray[index];
while (index < currentSize / 2) {
int leftChild = 2 * index + 1;
int rightChild = leftChild + 1;
if (rightChild < currentSize && heapArray[leftChild].compareTo(heapArray[rightChild]) < 0) {
largerChild = rightChild;
} else {
largerChild = leftChild;
}
if (top.compareTo(heapArray[largerChild]) >= 0) {
break;
}
heapArray[index] = heapArray[largerChild];
index = largerChild;
}
heapArray[index] = top;
}
}
public class HeapSort {
public static void main(String[] args) {
String[] array = {"apple", "banana", "orange", "pear", "peach"};
BinaryHeap heap = new BinaryHeap(array.length);
for (String s : array) {
heap.insert(s);
}
for (int i = 0; i < array.length; i++) {
array[i] = heap.remove();
}
for (String s : array) {
System.out.print(s + " ");
}
}
}
```
在这个示例中,我们首先创建了一个二叉堆类 `BinaryHeap`,包含堆的初始化、插入、删除等方法。然后,我们创建了一个字符串数组,并将其插入到堆中。最后,我们依次从堆中删除元素,并将其存储到原数组中,即可得到排序后的字符串数组。
阅读全文
相关推荐
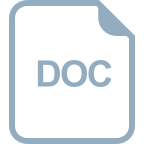
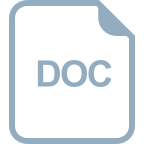
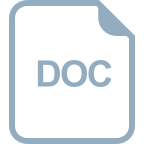
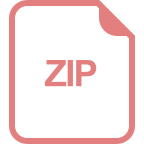
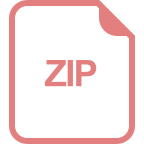
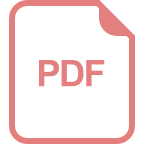
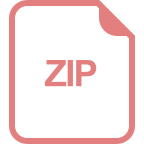
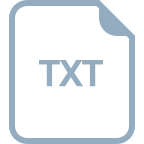
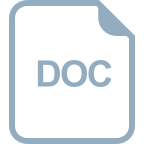
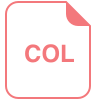
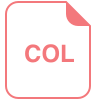
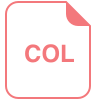
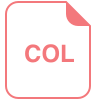
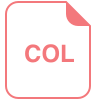
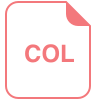
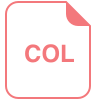
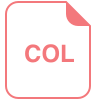
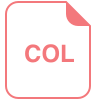
