java分布式文件上传,下载 代码
时间: 2024-01-01 13:08:44 浏览: 82
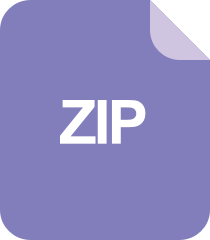
基于Java+Moosefs的分布式文件系统设计与实现(源码+文档)-Java-分布式文件系统.zip

以下是使用 Java NIO 实现分布式文件上传和下载的示例代码:
文件上传:
```java
import java.io.File;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileUploader {
public static void main(String[] args) throws IOException {
String host = "localhost";
int port = 9999;
String fileName = "test.txt";
Path filePath = Paths.get(fileName);
byte[] fileBytes = Files.readAllBytes(filePath);
ByteBuffer buffer = ByteBuffer.wrap(fileBytes);
SocketChannel channel = SocketChannel.open();
channel.connect(new InetSocketAddress(host, port));
channel.write(buffer);
channel.close();
}
}
```
文件下载:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileDownloader {
public static void main(String[] args) throws IOException {
String host = "localhost";
int port = 9999;
String fileName = "test.txt";
Path filePath = Paths.get(fileName);
FileOutputStream outputStream = new FileOutputStream(filePath.toFile());
SocketChannel channel = SocketChannel.open();
channel.connect(new InetSocketAddress(host, port));
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (channel.read(buffer) > 0) {
buffer.flip();
outputStream.write(buffer.array(), 0, buffer.limit());
buffer.clear();
}
outputStream.close();
channel.close();
}
}
```
注意事项:
1. 文件上传和下载的代码都需要指定主机地址(host)和端口号(port)。
2. 文件上传需要将文件读取到字节数组中,然后通过 SocketChannel 将字节数组写入网络中。
3. 文件下载需要将 SocketChannel 中的数据读取到 ByteBuffer 中,然后将 ByteBuffer 中的数据写入本地文件中。
4. 以上代码仅供参考,实际使用时需要根据具体需求进行修改和优化。
阅读全文
相关推荐
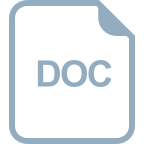
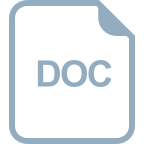
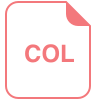

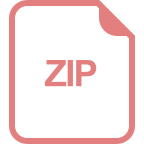
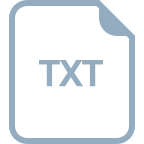
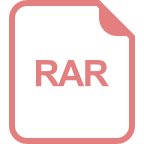
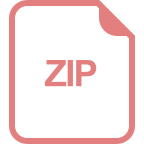
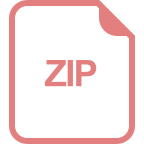
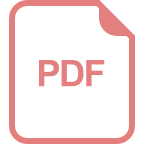
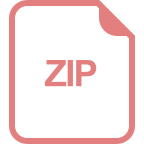
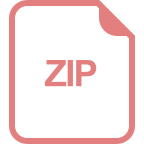
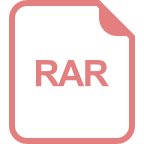
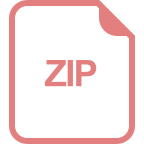
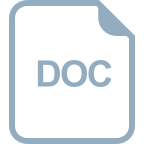
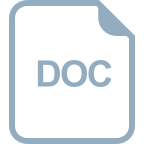
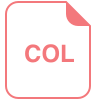