C语言贪吃蛇函数原型设计
时间: 2024-08-13 22:04:36 浏览: 99
在C语言中,贪吃蛇游戏的函数原型设计通常涉及到游戏循环、键盘输入处理、蛇和食物的位置管理等核心功能。虽然没有一个固定的函数原型,但我们可以将关键部分的函数进行设计。以下是一个简化的示例:
```c
// 定义蛇的位置数组
typedef struct {
int x;
int y;
} Snake;
// 定义食物结构
typedef struct {
int x;
int y;
} Food;
// 函数原型:初始化游戏
void init_game(Snake* snake, Food* food);
// 函数原型:处理用户输入
void handle_input(int key, Snake* snake);
// 函数原型:更新蛇的位置
void update_snake(Snake* snake, Food* food);
// 函数原型:检查碰撞
int check_collision(Snake* snake, Food* food);
// 函数原型:显示游戏画面
void draw_board(Snake* snake, Food* food);
// 主游戏循环
void game_loop();
// 清理资源的函数(可选)
void cleanup();
相关问题
c语言贪吃蛇代码ege
### C语言实现贪吃蛇游戏源码示例
以下是基于已有资料整理的一个完整的C语言贪吃蛇游戏代码实例:
#### 头文件 `Snake.h`
此头文件定义了游戏中所需的数据结构以及函数原型。
```c
#ifndef SNAKE_H
#define SNAKE_H
#include <stdio.h>
#include <stdlib.h>
#include <conio.h> /* _kbhit(), getch() */
#include <windows.h>
typedef struct {
COORD pos;
} FOOD;
typedef struct Node {
COORD coord;
struct Node* next;
} NODE, *PNODE;
typedef struct {
PNODE head;
PNODE tail;
int length;
char dir;
} SNAKE;
void InitFood(FOOD*);
void DrawMap();
void CreateNode(PNODE*, COORD);
void FreeList(PNODE);
int IsEatSelf(SNAKE*);
void MoveSnake(SNAKE*);
#endif //SNAKE_H
```
#### 主程序 `main.c`
这是整个项目的入口点,包含了初始化、循环处理输入和更新状态等功能。
```c
#include "Snake.h"
void GameStart(SNAKE* s) {
s->head = NULL;
s->tail = NULL;
s->length = 0;
s->dir = 'R';
}
void GameRun(SNAKE* s) {
FOOD food;
InitFood(&food);
while (1) {
if (_kbhit()) {
switch (getch()) {
case 'w': if (s->dir != 'D') s->dir = 'U'; break;
case 'a': if (s->dir != 'R') s->dir = 'L'; break;
case 'd': if (s->dir != 'L') s->dir = 'R'; break;
case 's': if (s->dir != 'U') s->dir = 'D'; break;
case 'q': exit(0);
}
}
system("cls");
DrawMap();
if (IsEatSelf(s)) {
printf("\nGame Over!\n");
Sleep(2000);
return;
}
MoveSnake(s);
if ((s->head)->coord.X == food.pos.X && (s->head)->coord.Y == food.pos.Y) {
CreateNode(&(s->tail), GetRandomCoord());
InitFood(&food);
}
}
}
void GameEnd(SNAKE* s) {
FreeList(s->head);
}
```
上述代码展示了如何创建一个简单的贪吃蛇游戏框架[^1]。通过`Snake.h`中的数据结构定义和支持函数声明,配合`main.c`里的具体逻辑实现了基本的游戏功能,包括地图绘制、方向控制、碰撞检测等操作[^3]。
阅读全文
相关推荐








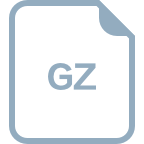








