for (const auto &det : Z) { Eigen::VectorXd a = x_ + K * (det - z_pre_); x_filter = x_filter + beta(i) * a; ++i; } x_filter = last_beta * x_ + x_filter; Eigen::MatrixXd P_temp = Eigen::MatrixXd(n_x_, n_x_); P_temp.fill(0.); for (int i = 0; i < Z.size() + 1; ++i) { Eigen::VectorXd a = Eigen::VectorXd(n_x_); a.setZero(); if (i == Z.size()) { a = x_; } else { a = x_ + K * (Z.at(i) - z_pre_); } P_temp = P_temp + beta(i) * (a * a.transpose() - x_filter * x_filter.transpose()); } x_ = x_filter; P_ -= (1 - last_beta) * K * S_ * K.transpose(); P_ += P_temp;
时间: 2024-04-28 12:23:03 浏览: 8
这段代码是一个卡尔曼滤波器的更新过程,其中更新了卡尔曼滤波器的状态向量x_和协方差矩阵P_。具体的更新过程如下:
1. 对于Z中的每个观测值det,计算卡尔曼增益K和状态向量a,然后更新x_filter(滤波后的状态向量)。
2. 计算P_temp(一个临时的协方差矩阵),其中beta是一个权重向量,用于加权每个状态向量的影响。对于每个状态向量a,计算出其对应的协方差矩阵,然后使用beta权重加权求和,得到P_temp。
3. 根据最终的滤波后状态向量x_filter和上一时刻的状态向量x_,以及最后一个观测值对应的beta值last_beta,计算出最终的状态向量x_。
4. 根据卡尔曼增益K和残差协方差矩阵S_,更新协方差矩阵P_。
总的来说,这段代码是用于对一个向量序列进行卡尔曼滤波的,其中涉及到了矩阵和向量的计算。
相关问题
Eigen::Tensor<uint8_t, 3, Eigen::RowMajor> crop_pointcloud( const Eigen::Tensor<Scalar, 3, Eigen::RowMajor> data_crop, Scalar x_o, Scalar y_o, Scalar x_i, Scalar y_i, Scalar R_o, Scalar R_i, Scalar z_critical) { const int range_z = data_crop.dimension(2); const Scalar K_o = R_o * R_o / range_z; const Scalar K_i = R_i * R_i / range_z; Eigen::Tensor<uint8_t, 3, Eigen::RowMajor> cropped_data = data_crop.cast<uint8_t>(); for (int z = 0; z < range_z; z++) { const Scalar r_o = std::sqrt(z * K_o); auto data_layer = cropped_data.chip(z, 2); const Scalar d_o = std::sqrt(x_o * x_o + y_o * y_o); const Scalar d_i = std::sqrt(x_i * x_i + y_i * y_i); const Scalar r_i = (z < z_critical) ? 0 : std::sqrt(z * K_i); data_layer = data_layer * ((d_o <= r_o) && (d_i > r_i)).template cast<uint8_t>(); } return cropped_data; } 参数改为int
Eigen::Tensor<uint8_t, 3, Eigen::RowMajor> crop_pointcloud(
const Eigen::Tensor<int, 3, Eigen::RowMajor> data_crop,
int x_o, int y_o, int x_i, int y_i,
int R_o, int R_i, int z_critical) {
const int range_z = data_crop.dimension(2);
const int K_o = R_o * R_o / range_z;
const int K_i = R_i * R_i / range_z;
Eigen::Tensor<uint8_t, 3, Eigen::RowMajor> cropped_data = data_crop.cast<uint8_t>();
for (int z = 0; z < range_z; z++) {
const int r_o = std::sqrt(z * K_o);
auto data_layer = cropped_data.chip(z, 2);
const int d_o = std::sqrt(x_o * x_o + y_o * y_o);
const int d_i = std::sqrt(x_i * x_i + y_i * y_i);
const int r_i = (z < z_critical) ? 0 : std::sqrt(z * K_i);
data_layer = data_layer * ((d_o <= r_o) && (d_i > r_i)).template cast<uint8_t>();
}
return cropped_data;
}
def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop 转Eigen,c++
此 Python 代码的主要作用是裁剪一个点云数据,其中包含了一些数学计算和条件判断。以下是一个可能的 C++ 实现:
```cpp
#include <cmath>
#include <Eigen/Core>
Eigen::ArrayXXd crop_pointcloud(
const Eigen::ArrayXXd& data_crop,
double x_o,
double y_o,
double x_i,
double y_i,
double R_o,
double R_i,
int z_critical)
{
int range_z = data_crop.cols();
double K_o = R_o * R_o / range_z;
double K_i = R_i * R_i / range_z;
Eigen::ArrayXXd result = data_crop;
for (int z = 0; z < range_z; ++z) {
double r_o = std::sqrt(z * K_o);
const auto& data_layer = data_crop.col(z);
double d_o = std::sqrt(x_o * x_o + y_o * y_o);
double d_i = std::sqrt(x_i * x_i + y_i * y_i);
double r_i = (z < z_critical) ? 0 : std::sqrt(z * K_i);
result.col(z) = (d_o <= r_o) && (d_i > r_i) ? data_layer : Eigen::ArrayXd::Zero(data_layer.rows());
}
return result;
}
```
需要注意的是,这里的 C++ 实现使用了 `Eigen::ArrayXXd` 类型来表示二维数组,其中 `Array` 表示支持向量化的运算,`XX` 表示支持动态大小。此外,由于 C++ 并没有内置的布尔数组类型,我们使用了 `Eigen::ArrayXd` 类型来表示只有一个元素的布尔数组。
相关推荐
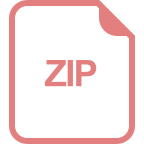
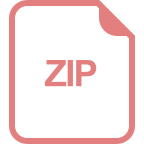
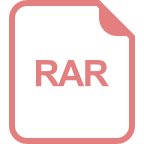












